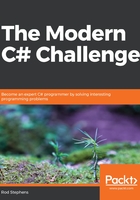
上QQ阅读APP看书,第一时间看更新
18. Perfect numbers
You can modify the preceding solution to find perfect numbers. Because you only need to calculate the sum of each value's divisors once, there's no need to pre-calculate those values and store them in an array. That wouldn't cost you much time, but it would use up a bunch of unnecessary memory.
The following code shows a method that finds perfect numbers:
// Find perfect numbers <= max.
private List<long> FindPerfectNumbers(long max)
{
// Look for perfect numbers.
List<long> values = new List<long>();
for (int value = 1; value <= max; value++)
{
long sum = GetProperDivisors(value).Sum();
if (value == sum) values.Add(value);
}
return values;
}
This method loops through the values that are less than the maximum. For each value, it calls the GetProperDivisors method, which is used in the previous solution, and calls Sum to add the divisors. If the sum equals the original number, the code adds the number to its list of perfect numbers.
Download the PerfectNumbers example solution to see additional details.