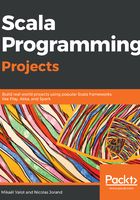
Types
We saw in the previous examples that Scala expressions have a type. For instance, the value 1 is of type Int, and the expression 1+1 is also of type Int. A type is a classification of data and provides a finite or infinite set of values. An expression of a given type can take any of its provided values.
Here are a few examples of types available in Scala:
- Int provides a finite set of values, which are all the integers between -231 and 231-1.
- Boolean provides a finite set of two values: true and false.
- Double provides a finite set of values: all the 64 bits and IEEE-754 floating point numbers.
- String provides an infinite set of values: all the sequence of characters are of an arbitrary length. For instance, "Hello World" or "Scala is great !".
A type determines the operations that can be performed on the data. For instance, you can use the + operator with two expressions of type Int or String, but not with expressions of type Boolean:
scala> val str = "Hello" + "World"
str: String = HelloWorld
scala> val i = 1 + 1
i: Int = 2
scala> val b = true + false
<console>:11: error: type mismatch;
found : Boolean(false)
When we attempt to use an operation on a type that does not support it, the Scala compiler complains of a type mismatch error.
An important feature of Scala is that it is a statically typed language. This means that the type of a variable or expression is known at compile time. The compiler will also check that you do not call an operation or function that is not legal for this type. This helps tremendously to reduce the number of bugs that can occur at runtime (when running a program).
As we saw earlier, the type of an expression can be specified explicitly with : followed by the name of the type, or in many cases, it can be automatically inferred by the compiler.
If you are not used to working with statically typed languages, you might get frustrated to have to fight with the compiler to make it accept your code, but you will gradually get more accustomed to the kind of errors thrown at you and how to resolve them. You will soon find that the compiler is not an enemy that prevents you from running your code; it is acting more like a good friend that shows you what logical errors you have made and gives you some indication on how to resolve them.
People coming from dynamically typed languages such as Python, or people coming from not-as-strongly statically typed language such as Java or C++, are often astonished to see that a Scala program that compiles has a much higher probability of being correct on the first run.
For instance, type val a = 3 in the Scala console, then move the cursor at the beginning of the a. You should see a light bulb icon. When you click on it, you will see a hint add type annotation to value definition. Click on it, and IntelliJ will add : Int after the a.
Your definition will become val a: Int = 3.