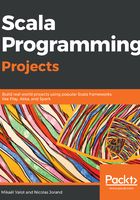
Refactoring the production code
In the TDD approach, it is common to refactor the code once we have passing tests. If our test coverage is good, we should not have any fear of changing the code, because any mishap should be flagged by a failing test. This is known as a Red-Green-Refactor cycle.
Change the body of futureCapital with the following code:
def futureCapital(interestRate: Double, nbOfMonths: Int, netIncome: Int, currentExpenses: Int, initialCapital: Double): Double = {
val monthlySavings = netIncome - currentExpenses
(0 until nbOfMonths).foldLeft(initialCapital)(
(accumulated, _) => accumulated * (1 + interestRate) +
monthlySavings)
}
Here, we have inlined the nextCapital function in the foldLeft call. In Scala, we can define an anonymous function using the syntax:
(param1, param2, ..., paramN) => function body.
We saw earlier that the month parameter in nextCapital was not used. In an anonymous function, it is a good practice to name any unused parameter with _. A parameter named _ cannot be used in the function body. If you try to replace the _ character with a name, IntelliJ will underline it. If you hover the mouse over it, you will see a popup stating Declaration is never used. You can then hit Alt + Enter and choose to remove the unused element to automatically change it back to _.