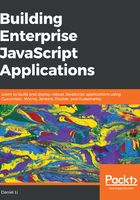
The env preset
However, in the previous approach, you have to manually keep track of which ECMAScript features you've used, and determine whether they are compatible with the version of Node.js you have installed on your machine. Babel provides a better alternative, the env preset, which is available as the @babel/preset-env package. This preset will use the kangax ECMAScript compatibility tables (kangax.github.io/compat-table/) to determine which features are unsupported by your environment, and download the appropriate Babel plugins.
This is great for our use case, because we don't want to transpile everything into ES5, only the import/export module syntax. Using the env preset will ensure that only the minimum number of transformations are made to our code.
In fact, if you go to the npmjs.com page for @babel/preset-es2017 or similar packages, you'll see that they have been deprecated in favor of the @babel/preset-env package. Therefore, we should remove the previous plugins and presets, and use the env preset instead:
$ yarn remove @babel/preset-es2017 @babel/plugin-syntax-object-rest-spread
$ yarn add @babel/preset-env --dev
Next, replace the content of our .babelrc with the following:
{
"presets": ["@babel/env"]
}
The API we are writing is intended to be run only on the server, using Node, so we should specify that in the configuration. We can specify the exact version of Node we want to support, but even better, we can ask Babel to detect it for us using the target option "node": "current".
So, replace .babelrc with the following:
{
"presets": [
["@babel/env", {
"targets": {
"node": "current"
}
}]
]
}
Great! Now we can continue writing in ES6. When we want to run our program, we can simply transpile it using Babel, and run the compiled script:
$ npx babel index.js -o compiled.js
$ node compiled.js
You should, once again, receive the 'Hello World!' text as the response when you send a GET request to localhost:8080.