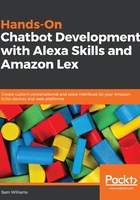
AWS CLI build script
These CLI commands are good, but typing this all out every time you want to upload a new Lambda version becomes annoying. We’re going to use a build script to automate these commands and add in a few extra features.
For this exact script to work, you need to have a folder structure as shown in the following screenshot. Each Lambda has a folder with the relevant files:

We will create a script that not only runs the basic AWS CLI commands, but also does extra checks, runs npm install, and echos out details about the progress. This script will be executed by running ./build lambda-folder.
Create a new file called build.sh in your lambdas folder. Alternatively, you can download this file from http://bit.ly/chatbot-ch2 and follow along to see how it works.
To start, we will check that exactly one parameter has been passed in the command. "$#" means the number of parameters and -ne 1 means not equal to 1:
if [ "$#" -ne 1 ]; then
echo "Usage : ./build.sh lambdaName";
exit 1;
fi
Next, we need to move into the folder for the selected Lambda and check that the folder exists:
lambda=${1%/}; // # Removes trailing slashes
echo "Deploying $lambda";
cd $lambda;
if [ $? -eq 0 ]; then
echo "...."
else
echo "Couldn't cd to directory $lambda. You may have mis-spelled the lambda/directory name";
exit 1
fi
We don't want to upload our Lambda without making sure that we've installed all of the dependencies, so we make sure to run npm install and check that it has been successful:
echo "npm installing...";
npm install
if [$? -eq 0 ]; then
echo "done";
else
echo "npm install failed";
exit 1;
fi
The last of the setup steps is to check that aws-cli is installed:
echo "Checking that aws-cli is installed"
which aws
if [ $? -eq 0 ]; then
echo "aws-cli is installed, continuing..."
else
echo "You need aws-cli to deploy this lambda. Google 'aws-cli install'"
exit 1
fi
With everything set up, we can create our new ZIP file. Before creating the new .zip file, we'll delete the old one. Creating a new one this time is a little bit more advanced than before. We exclude .git, .sh, and .zip files as well as excluding test folders and node_modules/aws-sdk from the file. We can exclude aws-sdk because it is already installed on all Lambda functions and we don't want to upload Git files, bash scripts, or other .zip files:
echo "removing old zip"
rm archive.zip;
echo "creating a new zip file"
zip archive.zip * -r -x .git/\* \*.sh tests/\* node_modules/aws-sdk/\* \*.zip
Now, all there is left to do is to upload it to AWS. We want to make this as easy as possible so we're going to try creating a new function. If that errors then we'll try updating the function. This could be done as a get and then create or update, but a failure to create is actually quicker than a get:
echo "Uploading $lambda to $region";
aws lambda create-function --function-name $lambda --runtime nodejs8.10 --role arn:aws:iam::095363550084:role/service-role/Dynamo --handler index.handler --zip-file fileb://index.zip --publish
if [ $? -eq 0 ]; then
echo "!! Create successful !!"
exit 1;
fi
aws lambda update-function-code --function-name $lambda --zip-file fileb://archive.zip --publish
if [ $? -eq 0 ]; then
echo "!! Update successful !!"
else
echo "Upload failed"
echo "If the error was a 400, check that there are no slashes in your lambda name"
echo "Lambda name = $lambda"
exit 1;
fi
To make the script executable, we need to change the permissions on the file. To do this, we run chmod +x ./build.sh.
Now, all you need to do is to navigate to the main folder where the Lambdas function resides and run ./build.sh example-lambda. If you have Lambdas folders nested in groups, then navigate into the group folders and run ../build.sh lambda-in-group.
If you want, you can move the build script to your home directory to make execution ~/build.sh lambda-function, which is only useful if you have Lambdas in separate folders or highly nested folders.
This script could be modified and expanded to include region-specific uploading, batch uploading multiple Lambda functions, Git integration, and lots more.