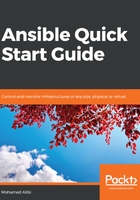
Ansible Docker container installation
Using Ansible on a container requires a container engine to be running. There are multiple choices for which container to use, the most famous ones being Docker, Kubernetes, and Red Hat OpenShift. In this book, we will only cover Docker. We need to have a Docker engine running on the machine that is going to host the Ansible Container. The information about Docker installation can be found in its official documentation at: https://docs.docker.com/install/. This covers a large number of operating systems.
Here, we will assume that the Docker engine is installed, and that the current user has been added to the Docker group so that they can manage the local Docker containers on the machine. You can also choose to build your own container by selecting any of the systems that you are familiar with for the source image. Make sure that you have all requirements installed. The following is an example of a basic Dockerfile on Linux Alpine, one of the lightest systems used on containers:
FROM alpine:3.7
RUN echo "#### Setting up the environment for the build dependencies ####" && \
set -x && apk --update add --virtual build-dependencies \
gcc musl-dev libffi-dev openssl-dev python-dev
RUN echo "#### Update the OS package index and tools ####" && \
apk update && apk upgrade
RUN echo "#### Setting up the build dependecies ####" && \
apk add --no-cache bash curl tar openssh-client \
sshpass git python py-boto py-dateutil py-httplib2 \
py-jinja2 py-paramiko py-pip py-yaml ca-certificates
RUN echo "#### Installing Python PyPI ####" && \
pip install pip==9.0.3 && \
pip install python-keyczar docker-py
RUN echo "#### Installing Ansible latest release and cleaning up ####" && \
pip install ansible –upgrade \
apk del build-dependencies && \
rm -rf /var/cache/apk/*
RUN echo "#### Initializing Ansible inventory with the localhost ####" && \
mkdir -p /etc/ansible/library /etc/ansible/roles /etc/ansible/lib /etc/ansible/ && \
echo "localhost" >> /etc/ansible/hosts
ENV HOME /home/ansible
ENV PATH /etc/ansible/bin:$PATH
ENV PYTHONPATH /etc/ansible/lib
ENV ANSIBLE_ROLES_PATH /etc/ansible/roles
ENV ANSIBLE_LIBRARY /etc/ansible/library
ENV ANSIBLE_SSH_PIPELINING True
ENV ANSIBLE_GATHERING smart
ENV ANSIBLE_HOST_KEY_CHECKING false
ENV ANSIBLE_RETRY_FILES_ENABLED false
RUN adduser -h $HOME ansible -D \
&& chown -R ansible:ansible $HOME
RUN echo "ansible ALL=(ALL) NOPASSWD: ALL" >> /etc/sudoers \
&& chmod 0440 /etc/sudoers
WORKDIR $HOME
USER ansible
ENTRYPOINT ["ansible"]
We then build the container using the build function on Docker:
docker build -t dockerhub-user/ansible .
The build might take some time to finish. We can then try and run our Ansible container in several different ways, depending on how we are going to use it. For example, we can verify the Ansible version on the container:
docker run --rm -it -v ~:/home/ansible dockerhub-user/ansible --version
We can also run a ping task:
docker run --rm -it -v ~:/home/ansible \
-v ~/.ssh/id_rsa:/ansible/.ssh/id_rsa \
-v ~/.ssh/id_rsa.pub:/ansible/.ssh/id_rsa.pub \
dockerhub-user/ansible -m ping 192.168.1.10
By changing the ENTRYPOINT of our Dockerfile code from [ansible] to [ansible-playbook], we can create a script that can use our container to work as if docker-playbook is installed. This will be explained further in Chapter 3, Ansible Inventory and Playbook. Create a script called ansible-playbook and add it to the PATH environmental variable with the following code:
#!/bin/bash
-v ~/.ssh/id_rsa:/ansible/.ssh/id_rsa \
-v ~/.ssh/id_rsa.pub:/ansible/.ssh/id_rsa.pub \
-v /var/log/ansible/ansible.log \
dockerhub-user/ansible "$@"
This script can be used as follows to execute a playbook on a specific host located in the inventory folder:
Ansibleplaybook play tasks.yml -i inventory/hosts