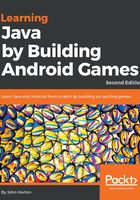
Setting up our objects with constructors
With all these private variables and their getters and setters, does it mean that we need a getter and a setter for every private variable? What about a class with lots of variables that need initializing at the start. Think about this code:
mySoldier.name mysoldier.type mySoldier.weapon mySoldier.regiment ...
Some of these variables might need getters and setters, but what if we just want to set things up when the object is first created, to make the object function correctly?
Surely, we don't need two methods (a getter and a setter) for each?
Fortunately, this is unnecessary. For solving this potential problem there is a special method called a constructor. We briefly mentioned the existence of a constructor when we discussed instantiating an object from a class. Let's take a look again. Here we create an object of type Soldier
and assign it to an object called mySoldier
.
Soldier mySoldier = new Soldier();
Nothing new here but look at the last part of that line of code.
...Soldier();
This looks suspiciously like a method call.
All along, we have been calling a special method called a constructor that has been created, behind the scenes, automatically, by the compiler.
However, and this is getting to the point now, like a method, we can override it which means we can do useful things to set up our new object before it is used. This next code shows how we could do this.
public Soldier(){ // Someone is creating a new Soldier object health = 200; // more setup here }
The constructor has a lot of syntactical similarities to a method. It can however only be called with the use of the new
keyword and it is created for us automatically by the compiler- unless we create our own like in the previous code.
Constructors have the following attributes:
- They have no return type
- They have the exact same name as the class
- They can have parameters
- They can be overloaded
One more piece of Java syntax which is useful to introduce at this point is the Java this
keyword.
The this
keyword is used when we want to be explicit about exactly which variables we are referring to. Look at this example constructor, again for a hypothetical variation of the Soldier
class.
public class Soldier{ String name; String type; int health; public Soldier(String name, String type, int health){ // Someone is creating a new Soldier object this.name = name; this.type = type; this.health = health; // more setup here } }
This time the constructor has a parameter for each of the variables we want to initialize.
Tip
A key point to note about constructors that can cause confusion is that once you have provided your own constructor, perhaps like the previous one, the default one (with no parameters) no longer exists.
Let's discuss this
a bit more.