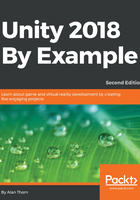
Counting coins
The coin collection game wouldn't really be much of a game if there were only one coin. The central idea is that a level should feature many coins, all of which the player should collect before a timer expires. Now, to know whether all coins have been collected, we'll need to know how many coins there are in total in the scene. After all, if we don't know how many coins there are, then we can't know if we've collected them all. So, our first task in scripting is to configure the Coin class so that we can know the total number of coins in the scene at any moment easily. Consider Code Sample 2.3, which adapts the Coin class to achieve this:
//------------------------- using UnityEngine; using System.Collections; //------------------------- public class Coin : MonoBehaviour { //------------------------- //Keeps track of total coin count in scene public static int CoinCount = 0; //------------------------- // Use this for initialization void Start () { //Object created, increment coin count ++Coin.CoinCount; } //------------------------- //Called when object is destroyed void OnDestroy() { //Decrement coin count --Coin.CoinCount; //Check remaining coins if(Coin.CoinCount <= 0) { //We have won } } //------------------------- } //-------------------------
Code Sample 2.3
The following points summarize the code sample:
- The Coin class maintains a static member variable, CoinCount, which, being static, is shared across all instances of the class. This variable keeps count of the total number of coins in the scene and each instance has access to it.
- The Start function is called once per Coin instance when the object is created in the Scene. For coins that are present when the scene begins, the Start event is called at scene startup. This function increments the CoinCount variable by one per instance, thus keeping count of all coins.
- The OnDestroy function is called once per instance when the object is destroyed. This decrements the CoinCount variable, reducing the count for each coin destroyed.
Altogether, Code Sample 2.3 maintains a CoinCount variable. In short, this variable allows us to always keep track of the total coin count. We can query it easily to determine how many coins remain. This is good, but is only the first step towards completing the coin collection functionality.