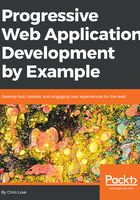
Your add to homescreen responsibilities
At Google I/O 2018, it was announced that Chrome on Android will no longer include an automated add to homescreen prompt. Instead, it is your responsibility to create the user experience. Ultimately, the Chrome team decided to fall more in line with how other browser vendors are crafting their experiences.
The manifest specification takes time to define skeleton rules and minimal requirements for the add to homescreen experience. Rather than limiting all browsers to the same rules, the specification defines instalability signals that can be used as part of the add to homescreen prompt algorithm.
The prompt sequence should honor a modicum of privacy considerations and wait for the document to be fully loaded before issuing a prompt. The process should also allow the user to inspect the application name, icon, start URL, origin, and other properties. It is also recommended that the end user be allowed to modify some of the values. For example, changing the application name on their homescreen.
This is where the beforeinstallprompt event comes into play. This is your hook to a proper signal to prompt the user to install your progressive web app.
This event triggers when heuristics are met to trigger an add to homescreen experience. But instead of a native or built-in prompt from Chrome, you are responsible to prompt the user at an appropriate time after this event triggers.
Why did they change this? I am not 100% certain, though personally I thought it was a good idea to help promote web app installs. But this is somewhat intrusive and does not fall in line with other best practices. For example, when we look at enabling push notifications later in this book, you should not automatically pester the visitor to enable notifications.
There should be a little courtship before asking for the next level of engagement. I hate to use this analogy, but it has become canonical at this point; you can't just walk up to every pretty girl and ask them to marry you. It is a much longer process, where mutual trust must be earned.
Asking a visitor to add your icon to their homescreen is not exactly the same as marriage, but is more like asking them to go steady or be exclusively dating.
To use the beforeinstallprompt event, add an event listener callback in your site's JavaScript:
var deferredPrompt; window.addEventListener('beforeinstallprompt', function (e) { // Prevent Chrome 67 and earlier from automatically showing the prompt e.preventDefault(); // Stash the event so it can be triggered later. deferredPrompt = e; showAddToHomeScreen(); });
There are a few moving parts I need to review. First is that the event object (e) has two unique properties, platforms, and userChoice. Platforms are arrays indicating if the user can install a native app or a progressive web app. The userChoice property resolves a promise indicating if the user chose to install the app or not.
The other piece used in this code is the deferredPrompt variable. This is declared outside the event handler, so it can be used at a later time, in this case within the showAddToHomeScreen logic.
The showAddToHomeScreen method is called as soon as the event fires in this example, but a better practice is to defer the action to an appropriate time. Think about a user in the middle of an important application task. A sudden prompt to install the app would be a confusing distraction. It would serve you and the user better if you deferred the prompt till the action completes.
The showAddToHomeScreen method displays a special overlay, asking the user to install the app:
function showAddToHomeScreen() { var a2hsBtn = document.querySelector(".ad2hs-prompt"); a2hsBtn.style.display = "flex"; a2hsBtn.addEventListener("click", addToHomeScreen);
}
I added a simple overlay to the 2048 application that slides up when made visible. Check out the following screenshot:

Once the prompt is accepted, the user is presented with the native add to homescreen prompt, as shown in the following screenshot:

Finally, the addToHomeScreen method utilizes the deferredPrompt variable that we saved a reference to in the beforeinstallprompt event handler. It calls the prompt method, which displays the built-in dialog that's pictured in the preceding screenshot:
function addToHomeScreen() { ... if (deferredPrompt) { // Show the prompt deferredPrompt.prompt(); // Wait for the user to respond to the prompt deferredPrompt.userChoice .then(function (choiceResult) { if (choiceResult.outcome === 'accepted') { console.log('User accepted the A2HS prompt'); } else { console.log('User dismissed the A2HS prompt'); } deferredPrompt = null; }); } }
The method then uses the userChoice method to perform tasks based on the choice. Here, I am just logging the choice to the console. You could persist a token indicating the state, or cascade additional logic to perform additional tasks.
I think this is a good opportunity to initiate a thank you or onboarding experience.
The 2048 application is a very simple add to homescreen experience. You can expand this functionality to educate the user or tell them the benefits of adding your app to their homescreen. Flipkart has a neat educational sequence that explains how to install the app and why they should. It is a good experience to model and one of the reasons why they have been successful in using progressive web apps.