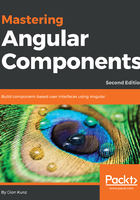
Composability
Composition is a special kind of reusability. You don't extend an existing component, but you create a new, larger component by composing many smaller components together into a system of components.
In OOP languages, composition is often used to get around the multiple inheritance issues that most OOP languages have. Subclass polymorphism is always great until you reach the point where your design does not match the latest requirements in your project. Let's look at a simple example that illustrates this problem.
You have a Fisher class and a Developer class, both of which hold specific behaviors. Now, you'd want to create a FishingDeveloper class that inherits from both Fisher and Developer. Unless you're using a language that supports multiple inheritance (such as C++, which does this to a certain extent), you will not be able to reuse this functionality using inheritance. There is no way to tell the language that your new class should inherit from both superclasses. Using composition, you can easily solve this problem. Instead of using inheritance, you're composing a new FishingDeveloper class that delegates all behavior to an internal Developer and Fisher instance:
interface IDeveloper {
code(): void;
}
interface IFisher {
fish(): void;
}
class Developer implements IDeveloper {
constructor(private name: string) {}
code(): void {
console.log(`${this.name} writes some code!`);
}
}
class Fisher implements IFisher {
constructor(private name: string) {}
fish(): void {
console.log(`${this.name} catches a big fish!`);
}
}
class FishingDeveloper implements IFisher, IDeveloper {
constructor(private name: string) {
this.name = name;
this.developerStuff = new Developer(name);
this.fisherStuff = new Fisher(name);
}
code(): void {
this.developerStuff.code();
}
fish(): void {
this.fisherStuff.fish();
}
}
var bob: FishingDeveloper = new FishingDeveloper('Bob');
bob.code();
bob.fish();
Experience has taught us that composition is probably the most efficient way to reuse code. In contrast to inheritance, decoration, and other approaches to gain reusability, composition is probably the least intrusive and the most flexible.
Recent versions of some languages also support a pattern called traits, that is, mixins. Traits allow you to reuse certain functionality and attributes from other classes in a way that is similar to multiple inheritance.
If we think about the concept of composition, it's nothing more than designing organisms. We have the two Developer and Fisher organisms, and we unify their behaviors into a single FishingDeveloper organism.