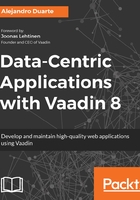
Servlets and UIs
A Vaadin application in its simplest form is a Servlet that delegates user interface logic to a UI implementation. The vaadin-server dependency includes the Servlet implementation: the VaadinServlet class. Let’s configure one.
Create a new directory with the name java inside the src/main directory.
Create a new package with the name packt.vaadin.datacentric.chapter01, and add a simple UI implementation inside this package:
public class VaadinUI extends UI {
@Override
protected void init(VaadinRequest vaadinRequest) {
setContent(new Label("Welcome to Data-Centric Applications with Vaadin 8!"));
}
}
Add a new WebConfig class to encapsulate everything related to web configuration, and define the VaadinServlet as an inner class:
public class WebConfig {
@WebServlet("/*")
@VaadinServletConfiguration(
ui = VaadinUI.class, productionMode = false)
public static class WebappVaadinServlet extends VaadinServlet {
}
}
The WebappVaadinServlet class must be public static to allow its instantiation by the Servlet Container. Notice how we are configuring /* as the servlet URL mapping using the @WebServlet annotation. This makes the application available at the root of the deployment path. Notice also how the @VaadinServletConfiguration annotation connects the Servlet to the UI implementation, the VaadinUI class we implemented in the previous step.