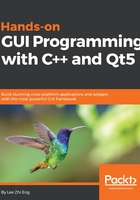
Basic Qt widgets
Now, we will take a look at the default set of widgets available in Qt Designer. You can actually create custom widgets by yourself, but that's an advanced topic which is out of the scope of this book. Let's take a look at the first two categories listed on the widget box—Layouts and Spacers:

Layouts and Spacers are not really something that you can directly observe, but they can affect the positions and orientations of your widgets:
- Vertical Layout: A vertical layout widget lays out widgets in a vertical column, from top to bottom.
- Horizontal Layout: A horizontal layout widget lays out widgets in a horizontal row, from left to right (or right to left for right-to-left languages).
- Grid Layout: A grid layout widget lays out widgets in a two-dimensional grid. Each widget can occupy more than one cell.
- Form Layout: A form layout widget lays out widgets in a two-column field style. Just as the name implies, this type of layout is best suited for forms of input widgets.
Layouts provided by Qt are very important for creating quality applications and are really powerful. Qt programs don't typically lay elements out using the fixed position because layouts allow dialogs and windows to be dynamically resized in a sensible manner while handling a varying length of text when it's localized in different languages. If you don't make use of layouts in your Qt programs, its UI may very look very different on different computers or devices, which in most cases will create an unpleasant user experience.
Next, let's take a look at the spacer widget. A spacer is a non-visible widget that pushes widgets along a specific direction until it reaches the limit of the layout container. Spacers must be used within a layout, otherwise they will not carry any effect.
There are two types of spacer, namely the Horizontal Spacer and Vertical Spacer:
- Horizontal Spacer: A horizontal spacer widget is a widget that occupies the space within a layout and pushes other widgets within the layout along a horizontal space.
- Vertical Spacer: A vertical spacer is similar to a horizontal spacer, except it pushes the widgets along the vertical space.
It's kind of hard to imagine how the Layouts and Spacers work without actually working with them. Don't worry about that, as we will be trying it out in a moment. One of the most powerful features of Qt Designer is that you can experiment with and test your layouts without have to change and compile your code after each change.
Besides Layouts and Spacers, there are a few more categories, namely Buttons, Item Views, Containers, Input Widgets, and Display Widgets. I won't go and explain every single one of them as their names are pretty much self-explanatory. You can also drag and drop the widget on the Form Editor to see what it does. Let's do it:
- Click and drag the Push Button widget from the Widget Box to the Form Editor, as shown in the following screenshot:

- Then, select the newly added Push Button widget, and you will see that all the information related to this particular widget is now appearing on the Properties Editor panel:

- You can change the properties of the widget, such as appearance, focus policy, tooltip, and so on programmatically in C++ code. Some properties can also be edited directly in the Form Editor. Let's double-click on the Push Button and change the text of the button, and then resize the button by dragging its edge:

- Once you're done with that, let's drag and drop a Horizontal Layout to the Form Editor. Then, drag the Push Button to the newly added layout. You will now see that the button automatically fits into the layout:

- By default, the main window does not carry any layout effect, and therefore the widgets will stay where they were originally placed, even when the window is being resized, which does not look very good. To add a layout effect to the main window, right-click on the window in the Form Editor, select Lay out, and finally select Lay Out Vertically. You will now see the Horizontal Layout widget we added previously is now automatically expanding to fit the entire window. This is the correct behavior of a layout in Qt:

- Next, we can play around with the spacer and see what effect it has. We will drag and drop a Vertical Spacer to the top of the layout containing the Push Button, and then we'll place two Horizontal Spacers on both sides of the button, within its layout:

The spacers will push all of the widgets located on both of their ends and occupy the space itself. In this example, the Submit button will always stay at the bottom of the window and keep its middle position, regardless of the size of the window. This makes the GUI look good, even on different screen sizes.
Ever since we added the spacers to the window, our Push Button has been squeezed to its minimum size. Let's enlarge the button by setting its minimumSize property to 120 x 40, and you'll see that the button appears a lot bigger now:

- After that, let's add a Form Layout above the layout of the Push Button and a Vertical Spacer below it. You will now see that the Form Layout is really thin because it has been squeezed by the Vertical Spacers we placed earlier onto the main window, which can be troublesome when you want to drag and drop a widget into the Form Layout. To solve this problem, temporarily set the layoutTopMargin property to 20 or higher:

- Then, drag and drop two Labels to the left side of the Form Layout and two Line Edits to its right side. Double click on both of the labels and change their display texts to Username: and Password:, respectively. Once you're done with that, set the layoutTopMargin property of the Form Layout back to 0:

Currently, the GUI looks pretty great, but the Form Layout is now occupying the entire spacing in the middle, which is not very pleasant when the main window is maximized. To keep the form compact, we'll do the following steps, which are a little tricky:
- First, drag and drop a Horizontal Layout above the form, and set its layoutTopMargin and layoutBottomMargin to 20 so that the widgets that we place in it, later on, are not too close to the Submit button. Next, drag and drop the entire Form Layout, which we placed earlier into the Horizontal Layout. Then, place Horizontal Spacers on both sides of the form to keep it centered. The following screenshot illustrates these steps:

- After that, we can make further adjustments to the GUI to make it look tidy before we proceed to the next section, where we will be customizing the widgets' style. Let's start off by setting the minimumSize property of the two Line Edit widgets to 150 x 25. Then, set the layoutLeftMargin, layoutRightMargin, layoutTopMargin, and layoutBottomMargin properties of the Form Layout to 25. The reason why we want to do this is that we will be adding an outline to the Form Layout in the following section.
- Since the Push Button is now way too distanced from the Form Layout, let's set the layoutBottomMargin property of the Horizontal Layout, which sets the Form Layout to 0. This will make the Push Button move slightly above and closer to the Form Layout. After that, we'll adjust the size of the Push Button to make it align with the Form Layout. Let's set the minimumSize property of the Push Button to 260 x 35, and we're done!:

You can also preview your GUI without building your program by going to Tools | Form Editor | Preview. Qt Designer is a very handy tool when it comes to designing sleek GUIs for Qt programs without a steep learning curve. In the following section, we will learn how to customize the appearance of the widgets using Qt Style Sheets.