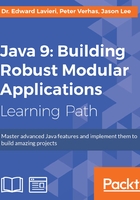
Compiling your application
Your next step is to compile your application using JDK 9's javac. This is important, even if your app works fine on JDK 9. You might not receive compiler errors, but watch for warnings too. Here are the most common reasons your applications might not compile with JDK 9, assuming they compiled fine prior to Java 9.
First, as indicated earlier in this chapter, most of the JDK 9 internal APIs are not accessible by default. Your indication will be an IllegalAccessErrors error at runtime or compile time. You will need to update your code so that you are using accessible APIs.
A second reason your pre-Java 9 applications might not compile with JDK 9 is if you use the underscore character as a single character identifier. According to Oracle, this practice generates a warning in Java 8 and an error in Java 9. Let's look at an example. The following Java class instantiates an Object named _ and prints a singular message to the console:
public class Underscore
{
public static void main(String[] args)
{
Object _ = new Object();
System.out.println("This ran successfully.");
}
}
When we compile this program with Java 8, we receive a warning that use of '_' as an identifier might not be supported in releases after Java SE 8:

As you can see in the following screenshot, that is just a warning and the application runs fine:

Now, let's try compiling the same class using JDK 9:

As you can see, use of the underscore as a single character identifier still only resulted in a warning and not an error. The application ran successfully. This test was run when JDK 9 was still in early release. It is assumed that running this test once JDK 9 has been officially released will result in an error instead of just a warning. The error that would likely be thrown is as follows:
Underscore.java:2: error: as of release 9, '_' is a keyword, and may not be used as a legal identifier.
Even if this issue is not resolved with the formal release of JDK 9, use of an underscore as a single character identifier is not good programming practice, so you should steer away from using it.
A third potential reason for your pre-Java 9 programmed application not to compile with JDK 9 is if you are using the -source and -target compiler options. Let's take a look at the -source and -target compiler options pre-Java 9 and with Java 9.