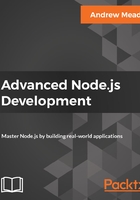
Querying users collection
To get started, let's head into Robomongo, open up the Users collection, and take a look at all the documents we have inside of there. We currently have five. If you don't have the exact same number or yours are a little different, that's fine. I'm going to highlight them, right-click them, and click Expand Recursively. This is going to show me all of the key-value pairs for each document:

Currently, aside from the ID, they're all identical. The name's Andrew, the age is 25, and the location is Philadelphia. I'm going to tweak the name property for two of them. I'm going to right-click the first document, and change the name to something like Jen. Then, I'll go ahead and do the same thing for the second document. I'm going to edit that document and change the name from Andrew to Mike. Now I have one document with a name of Jen, one with Mike, and three with Andrew.
We're going to query our users, looking for all of the users with the name equal to the name that you provided in the script. In this case, I'm going to try to query for all documents in the Users collection where the name is Andrew. Then, I'm going to print them into the screen, and I will expect to get three back. The two with the names Jen and Mike should not show up.
The first thing we need to do is fetch from the collection. This is going to be the Users collection as opposed to the Todos collection we've used in this chapter. In the db.collection, we're looking for the Users collection and now we're going to go ahead and call find, passing in our query. We want a query, fetching all documents where the name is equal to the string Andrew.
db.collection('Users').find({name: 'Andrew'})
This is going to return the cursor. In order to actually get the documents, we have to call toArray. We now have a promise; we can attach a then call onto toArray to do something with the docs. The documents are going to come back as the first argument in our success handler, and right inside of the function itself we can print the docs to the screen. I'm going to go ahead and use console.log(JSON.stringify()), passing in our three classic arguments: the object itself, docs, undefined, and 2 for formatting:
db.collection('Users').find({name: 'Andrew'}).toArray().then((docs) => { console.log(JSON.stringify(docs, undefined, 2)); });
With this in place, we have now done. We have a query, and it should work. We can test it by running it from the Terminal. Inside the Terminal, I'm going to go ahead and shut down the previous connection and rerun the script:

When I do this, I get my three documents back. All of them have a name equal to Andrew, which is correct because of the query we set up. Notice the documents with a name equal to Mike or Jen are nowhere to be found.
We now know how to insert and query data from the database. Up next, we're going to take a look at how we can remove and update documents.