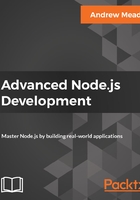
Saving the instance to the database
Creating a new instance alone does not actually update the MongoDB database. What we need to do is call a method on newTodo. This is going to be newTodo.save. The newTodo.save method is going to be responsible for actually saving text to the MongoDB database. Now, save returning a promise, which means we can tack on a then call and add a few callbacks.
newTodo.save().then((doc) => { }, (e) => { });
We'll add the callbacks for when the data either gets saved or when an error occurs because it can't save for some reason. Maybe the connection failed, or maybe the model is not valid. Either way, for now we'll just print a little string, console.log(Unable to save todo). Up above, in the success callback, we're actually going to get that Todo. I can call the argument doc, and I can print it to the screen, console.log. I'll print a little message first: Saved todo, and the second argument will be the actual document:
newTodo.save().then((doc) => { console.log('Saved todo', doc); }, (e) => { console.log('Unable to save todo'); });
We've configured Mongoose, connecting to the MongoDB database; we've created a model, specifying the attributes we want Todos to have; we created a new Todo; and finally, we saved it to the database.