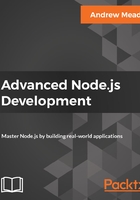
Creating a second Todo model
We have one Todo created using our Mongoose model. What I want you to do is make a second one, filling out all three values. This means you're going to make a new Todo with a text value, a completed Boolean; go ahead and set that to true; and a completedAt timestamp, which you can set to any number you like. Then, I want you to go ahead and save it; print it to the screen if it saves successfully; print an error if it saves poorly. Then, finally, run it.
The first thing I would have done is made a new variable down below. I'm going to make a variable called otherTodo, setting it equal to a new instance of the Todo model.
var otherTodo = new Todo ({
});
From here, we can pass in our one argument, which is going to be the object, and we can specify all of these values. I can set text equal to whatever I like, for example, Feed the cat. I can set the completed value equal to true, and I can set completedAt equal to any number. Anything lower than 0, like -1, is going to go backwards from 1970, which is where 0 is. Anything positive is going to be where we're at, and we'll talk about time-stamps more later. For now, I'm going to go with something like 123, which would basically be two minutes into the year 1970.
var otherTodo = new Todo ({ text: 'Feed the cat', completed: true, completedAt: 123 });
With this in place, we now just need to call save. I'm going to call otherTodo.save. This is what's actually going to write to the MongoDB database. I am going to tack on a then callback, because I do want to do something once the save is complete. If the save method worked, we're going to get our doc, and I'm going to print it to the screen. I'm going to use that pretty-print system we talked about earlier, JSON.stringify, passing in the actual object, undefined, and 2.
var otherTodo = new Todo ({ text: 'Feed the cat', completed: true, completedAt: 123 }); otherTodo.save().then((doc) => { console.log(JSON.stringify(doc, undefined, 2));
})
You don't need to do this; you can print it in any way you like. Next up, I'm going to print a little message if things go poorly: console.log('Unable to save', e). It'll pass along that error object, so if someone's reading the logs, they can see exactly why the call failed:
otherTodo.save().then((doc) => { console.log(JSON.stringify(doc, undefined, 2)); }, (e) => { console.log('Unable to save', e); });
With this in place, we can now comment out that first Todo. This is going to prevent another one from being created, and we can rerun the script, running our brand-new Todo creation calls. In the Terminal, I'm going to shut down the old connection and start up a new one. This is going to create a brand-new Todo, and we have it right here:

The text property equals Feed the cat. The completed property sets to the Boolean true; notice there's no quotes around it. The completedAt equals the number 123; once again, no quotes. I can also go into Robomongo to confirm this. I'm going to refetch the Todos collection, and now we have two Todos:

On the right-hand side of the Values column, you'll also notice the Type column. Here, we have int32 for completedAt and the __v property. The completed property is a Boolean, text is a String, and the _id is an ObjectId type.
There's a lot of useful information hidden inside of Robomongo. If you want something, they most likely have it built in. That's it for this one. We now know how to use Mongoose to make a connection, create a model, and finally save that model to the database.