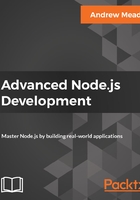
Customizing the Todo text property
To kick things off, let's customize how Mongoose treats our text property. Currently, we tell Mongoose that we want it to be a string, but we don't have any validators. One of the first things we can do for the text property is set required equal to true.
var Todo = mongoose.model('Todo', {
text: {
type: String,
required: true
},
When you set required equal to true, the value must exist, so if I were to try to save this Todo it would fail. And we can prove this. We can save the file, head over to the Terminal, shut things down, and restart it:

We get an unreadable error message. We'll dive into this in a second, but for now all you need to know is that we're getting a validation error: Todo validation failed, and that is fantastic.
Now, aside from just making sure the text property exists, we can also set up some custom validators. For strings, for example, we have a minlength validator, which is great. You shouldn't be able to create a Todo whose text is an empty string. We can set minlength equal to the minimum length, which we're is going to be 1 in this case:
var Todo = mongoose.model('Todo', { text: { type: String, required: true, minlength: 1 },
Now, even if we do provide a text property in the otherTodo function, let's say we set text equal to an empty string:
var otherTodo = new Todo ({ text: '' });
It's still going to fail. It is indeed there but it does not pass the minlength validator, where the minlength validator must be 1. I can save the server file, restart things over in the Terminal, and we still get a failure.
Now aside from required and minlength, there are a couple other utilities that are around in the docs. One good example is something called trim. It's fantastic for strings. Essentially, trim trims off any white space in the beginning or end of your value. If I set trim equal to true, like this:
var Todo = mongoose.model('Todo', { text: { type: String, required: true, minlength: 1,
trim: true },
It's going to remove any leading or trailing white space. So if I try to create a Todo whose text property is just a bunch of spaces, it's still going to fail:
var otherTodo = new Todo ({ text: ' ' });
The trim property is going to remove all of the leading and trailing spaces, leaving an empty string, and if I rerun things, we still get a failure. The text field is invalid. If we do provide a valid value, things are going to work as expected. Right in the middle of all of the spaces in otherTodo, I'm going to provide a real Todo value, which is going to be Edit this video:
var otherTodo = new Todo ({ text: ' Edit this video ' });
When we try to save this Todo, the first thing that's going to happen is the spaces in the beginning and the end of the string are going to get trimmed. Then, it's going to validate that this string has a minimum length of 1, which it does, and finally, it will save the Todo to the database. I'm going to go ahead and save server.js, restart our script, and this time around we get our Todo:

The Edit this video text shows up as the text property. Those leading and trailing spaces have been removed, which is fantastic. Using just three properties, we were able to configure our text property, setting up some validation. Now, we can do similar stuff for completed.