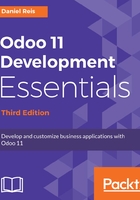
Adding automated tests
Programming best practices include having automated tests for your code. This is even more important for dynamic languages such as Python. Since there is no compilation step, you can't be sure there are no syntactic errors until the code is actually run by the interpreter. A good editor can help us spot these problems ahead of time, but can't help us ensure the code performs as intended like automated tests can.
Odoo supports two ways to describe tests: either using YAML data files or using Python code, based on the Unittest library. YAML tests are a legacy from older versions and are not recommended. We prefer using Python tests and will add a basic test case to our module.
The test code files should have a name starting with test_ and should be imported from tests/__init__.py. But the tests directory (also a Python submodule) should not be imported from the module's top __init__.py, since it will be automatically discovered and loaded only when tests are executed.
Tests must be placed in the tests/ subdirectory. Add a tests/__init__.py file with the following:
from . import test_todo
Now add the actual test code, available in the tests/test_todo.py file:
from odoo.tests.common import TransactionCase class TestTodo(TransactionCase): def test_create(self): "Create a simple Todo" Todo = self.env['todo.task'] task = Todo.create({'name': 'Test Task'}) self.assertEqual(task.is_done, False)
This adds a simple test case to create a new To-Do task and verifies that the Is Done? field has the correct default value.
Now we want to run our tests. This is done by adding the --test-enable option while installing or upgrading the module:
$ ./odoo-bin -d todo -u todo_app --test-enable
The Odoo server will look for a tests/ subdirectory in the upgraded modules and will run them. If any of the tests fail, the server log will show that.