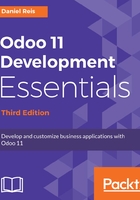
Testing access security
In fact, our tests should be failing right now due to the missing access rules. They aren't because they are done with the Admin user. In databases with demo data enabled, we also make available a Demo user, with "typical" backend access. We should change the tests so that they are performed using the Demo user.
We can modify our tests to take this into account. Edit the tests/test_todo.py file to add a setUp method:
def setUp(self, *args, **kwargs): result = super(TestTodo, self).setUp(*args, **kwargs) user_demo = self.env.ref('base.user_demo') self.env= self.env(user=user_demo) return result
This first instruction calls the setUp code of the parent class. The next ones change the environment used to run the tests, self.env, to a new one using the Demo user. No further changes are needed for the tests we already wrote.
We should also add a test case to make sure that users can see only their own tasks. To do this, first add an additional import at the top:
from odoo.exceptions import AccessError
Next, add an additional method to the test class:
def test_record_rule(self): "Test per user record rules" Todo = self.env['todo.task'] task = Todo.sudo().create({'name': 'Admin Task'}) with self.assertRaises(AccessError): Todo.browse([task.id]).name
Since our env method is now using the Demo user, we use the sudo() method to change the context to the admin user. We then use it to create a task that should not be accessible to the Demo user.
When trying to access this task data, we expect an AccessError exception to be raised.
If we run the tests now, they should fail, so let's take care of that.