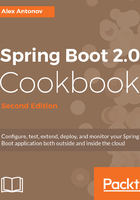
Getting ready
In our previous example, we created the basic application that will execute a command-line runner by printing a message in the logs. Let's enhance this application by adding a connection to a database.
Earlier, we already added the necessary jdbc and data-jpa starters as well as an H2 database dependency to our build file. Now we will configure an in-memory instance of the H2 database.
To demonstrate the fact that just by including the H2 dependency in the classpath, we will automatically get a default database, let's modify our StartupRunner.java file to look as follows:
public class StartupRunner implements CommandLineRunner { protected final Log logger = LogFactory.getLog(getClass()); @Autowired private DataSource ds; @Override public void run(String... args) throws Exception { logger.info("DataSource: "+ds.toString()); } }
Now, if we proceed with the running of our application, we will see the name of the datasource printed in the log, as follows:
2017-12-16 21:46:22.067 com.example.bookpub.StartupRunner
:DataSource: org.apache.tomcat.jdbc.pool.DataSource@4... {...driverClassName=org.h2.Driver; ... }
So, under the hood, Spring Boot recognized that we've autowired a DataSource bean dependency and automatically created one initializing the in-memory H2 datastore. This is all well and good, but probably not all too useful beyond an early prototyping phase or for the purpose of testing. Who would want a database that goes away with all the data as soon as your application shuts down and you have to start with a clean slate every time you restart the application?