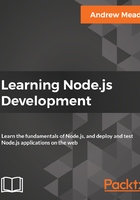
Working with fetchNotes
First up, let's do fetchNotes, which will need the following try-catch block.
I'll actually cut it out of addNote and paste it in the fetchNotes function, as shown here:
var fetchNotes = () => {
try{
var notesString = fs.readFileSync('notes-data.json');
notes = JSON.parse(notesString);
} catch (e) {
}
};
This alone is not enough, because currently we don't return anything from the function. What we want to do is to return the notes. This means that instead of saving the result from JSON.parse onto the notes variable, which we haven't defined, we'll simply return it to the calling function, as shown here:
var fetchNotes = () => {
try{
var notesString = fs.readFileSync('notes-data.json');
return JSON.parse(notesString);
} catch (e) {
}
};
So, if I call fetchNotes in the addNote function, shown as follows, I will get the notes array because of the return statement in the preceding code.
Now, if there are no notes, maybe there's no file at all; or there is a file, but the data isn't JSON, we can return an empty array. We'll add a return statement inside of catch, as shown in the following code block, because remember, catch runs if anything inside try fails:
var fetchNotes = () => {
try{
var notesString = fs.readFileSync('notes-data.json');
return JSON.parse(notesString);
} catch (e) {
return [];
}
};
Now, this lets us simplify addNote even further. We can remove the empty space and we can take the array that we set on the notes variable and remove it and instead call fetchNotes, as shown here:
var addNote = (title, body) => {
var notes = fetchNotes();
var note = {
title,
body
};
With this in place, we now have the exact same functionality we had before, but we have a reusable function, fetchNotes, which we can use in the addNote function to handle the other commands that our app will support.
Instead of copying code and having it in multiple places in your file, we've broken it into one place. If we ever want to change how this functionality works, whether we want to change the filename or some of the logic such as the try-catch block, we can change it once instead of having to change it in every function we have.