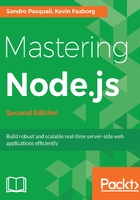
Duplex streams
A duplex stream is both readable and writeable. For instance, a TCP server created in Node exposes a socket that can be both read from, and written to:
const stream = require("stream");
const net = require("net");
net.createServer(socket => {
socket.write("Go ahead and type something!");
socket.setEncoding("utf8");
socket.on("readable", function() {
process.stdout.write(this.read())
});
})
.listen(8080);
When executed, this code will create a TCP server that can be connected to via Telnet:
telnet 127.0.0.1 8080
Start the server in one terminal window, open a separate terminal, and connect to the server via telnet. Upon connection, the connecting terminal will print out Go ahead and type something!—writing to the socket. Any text entered in the connecting terminal (after hitting ENTER) will be echoed to the stdout of the terminal running the TCP server (reading from the socket), creating a sort of chat application.
This implementation of a bidirectional (duplex) communication protocol demonstrates clearly how independent processes can form the nodes of a complex and responsive application, whether communicating across a network or within the scope of a single process.
The options sent when constructing a Duplex instance merge those sent to Readable and Writable streams, with no additional parameters. Indeed, this stream type simply assumes both roles, and the rules for interacting with it follow the rules for the interactive mode being used.
As a Duplex stream assumes both read and write roles, any implementation is required to implement both _write and _read methods, again following the standard implementation details given for the relevant stream type.