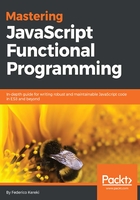
Functions as First Class Objects
Saying that functions are first class objects (also: first class citizens) means that you can do everything with functions, that you can do with other objects. For example, you can store a function in a variable, you can pass it to a function, you can print it out, and so on. This is really the key to doing FP: we will often be passing functions as parameters (to other functions) or returning a function as the result of a function call.
If you have been doing async Ajax calls, you have already been using this feature: a callback is a function that will be called after the Ajax call finishes and is passed as a parameter. Using jQuery, you could write something like:
$.get("some/url", someData, function(result, status) {
// check status, and do something
// with the result
});
The $.get() function receives a callback function as a parameter, and calls it after the result is obtained.
This is better solved, in a more modern way, by using promises or async/await, but for, but for the sake of our example, the older way is enough. We'll be getting back to promises, though, in section Building Better Containers, of chapter 12, Building Better Containers – Functional Data Types, when we discuss Monads; in particular, see section Unexpected Monads: Promises.
Since functions can be stored in variables, you could also write:
var doSomething = function(result, status) {
// check status, and do something
// with the result
};
$.get("some/url", someData, doSomething);
We'll be seeing more examples of this in Chapter 6, Producing Functions – Higher–Order Functions, when we consider Higher–Order Functions.