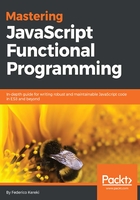
上QQ阅读APP看书,第一时间看更新
Solution #3 - remove the handler
We may go for a lateral kind of solution, and instead of having the function avoid repeated clicks, we might just remove the possibility of clicking altogether:
function billTheUser(some, sales, data) {
document.getElementById("billButton").onclick = null;
window.alert("Billing the user...");
// actually bill the user
}
This solution also has some problems:
- The code is tightly coupled to the button, so you won't be able to reuse it elsewhere
- You must remember to reset the handler, otherwise the user won't be able to make a second buy
- Testing will also be harder, because you'll have to provide some DOM elements
We can enhance this solution a bit, and avoid coupling the function to the button, by providing the latter's ID as an extra argument in the call. (This idea can also be applied to some of the following solutions.) The HTML part would be:
<button
id="billButton"
onclick="billTheUser('billButton', some, sales, data)"
>
Bill me
</button>;
(note the extra argument) and the called function would be:
function billTheUser(buttonId, some, sales, data) {
document.getElementById(buttonId).onclick = null;
window.alert("Billing the user...");
// actually bill the user
}
This solution is somewhat better. But, in essence, we are still using a global element: not a variable, but the onclick value. So, despite the enhancement, this isn't a very good solution either. Let's move on.