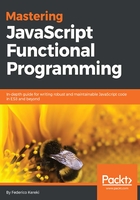
Adding missing functions
This idea of defining a function on the run, also allows us to write polyfills that provide otherwise missing functions. For example, let's say that instead of writing code like:
if (currentName.indexOf("Mr.") !== -1) {
// it's a man
...
}
You'd very much prefer using the newer, clearer way, and just write:
if (currentName.includes("Mr.")) {
// it's a man
...
}
What happens if your browser doesn't provide .includes()? Once again, we can define the appropriate function on the run, but only if needed. If .includes() is available, you need do nothing, but if it is missing, you then define a polyfill that will provide the very same workings.
You can find polyfills for many modern JS features at Mozilla's developer site. For example, the polyfill we used for includes was taken directly from https://developer.mozilla.org/en/docs/Web/JavaScript/Reference/Global_Objects/String/includes.
if (!String.prototype.includes) {
String.prototype.includes = function(search, start) {
"use strict";
if (typeof start !== "number") {
start = 0;
}
if (start + search.length > this.length) {
return false;
} else {
return this.indexOf(search, start) !== -1;
}
};
}
When this code runs, it checks whether the String prototype already has the includes method. If not, it assigns a function to it that does the same job, so from that point onward, you'll be able to use .includes() without further worries.
Directly modifying a standard type's prototype object is usually frowned upon, because in essence, it's equivalent to using a global variable, and thus prone to errors. However, in this case, writing a polyfill for a well established and known function, is quite unlikely to provoke any conflicts.
Finally, if you happened to think that the Ajax example shown previously was old hat, consider this: if you want to use the more modern fetch() way of calling services, you will also find that not all modern browsers support it (check http://caniuse.com/#search=fetch to verify that) and you'll have to use a polyfill too, such as the one at https://github.com/github/fetch . Study the code, and you'll see it basically uses the same method as described previously, to see if a polyfill is needed, and to create it.