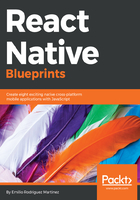
上QQ阅读APP看书,第一时间看更新
Setting up the store
After understanding how MobX works, we are ready to create our store:
/*** src/store.js ** */
import { observable } from 'mobx';
import { AsyncStorage } from 'react-native';
class Store {
@observable feeds;
@observable selectedFeed;
@observable selectedEntry;
constructor() {
AsyncStorage.getItem('@feeds').then(sFeeds => {
this.feeds = JSON.parse(sFeeds) || [];
});
}
_persistFeeds() {
AsyncStorage.setItem('@feeds', JSON.stringify(this.feeds));
}
addFeed(url, feed) {
this.feeds.push({
url,
entry: feed.entry,
title: feed.title,
updated: feed.updated,
});
this._persistFeeds();
}
removeFeed(url) {
this.feeds = this.feeds.filter(f => f.url !== url);
this._persistFeeds();
}
selectFeed(feed) {
this.selectedFeed = feed;
}
selectEntry(entry) {
this.selectedEntry = entry;
}
}
const store = new Store();
export default store;
We have already seen the basic structure of this file in the MobX section of this chapter. Now, we will add some methods to modify the list of feeds and to select a specific feed/entry when the user taps on them in our app's listings for feeds/entries.
We are also making use of AsyncStorage to persist the list of feeds every time it is modified by either addFeed or removeFeed.