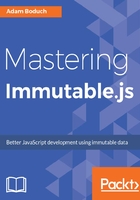
上QQ阅读APP看书,第一时间看更新
Chaining collection mutation methods
You've seen how you can chain collection insertion methods together and that there are two types of collection mutation method. Since each of these methods is a persistent change, they return a new collection, which means that you can chain together complex mutation behavior:
const myList = List.of(1, 2, 3);
const myMap = Map.of('one', 1, 'two', 2);
const myChangedList = myList
.push(4)
.set(3, 5)
.update(3, n => n + 5);
const myChangedMap = myMap
.set('three', 3)
.set('three', 5)
.update('three', t => t + 5);
console.log('myList', myList.toJS());
// -> myList [ 1, 2, 3 ]
console.log('myMap', myMap.toJS());
// -> myMap { one: 1, two: 2 }
console.log('myChangedList', myChangedList.toJS());
// -> myChangedList [ 1, 2, 3, 10 ]
console.log('myChangedMap', myChangedMap.toJS());
// -> myChangedMap { one: 1, two: 2, three: 10 }
Here, we're mutating a list and a map by chaining together insertion methods and mutation methods. A key design trait of the Immutable.js API is that the list methods and the map methods are the same. The obvious difference is push() versus set() for inserting items into lists and maps.