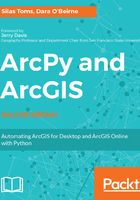
If/Elif/Else statements
Conditional statements, called if...else statements in Python, are another programming language standard. They are used when evaluating data; when certain conditions are met, one action will be taken (the initial if statement); if another condition is met, another action is taken (this is an elif statement), and if the data does not meet the condition, a final action is assigned to deal with those cases (the else statement). They are similar to a conditional in an SQL statement used with the Select Tool in ArcToolbox. Here is an example using an if...else statement to evaluate data in a list. In the example, within the for loop, the modulo operator % produces the remainder of a division operation. The if condition checks for no remainder when divided in half, a elif condition looks for remainder of two when divided by three, and the else condition catches any other result, as shown:
>>> data = [1,2,3,4,5,6,7]
>>> for val in data:
if val % 2 == 0:
print val,"no remainder"
elif val % 3 == 2:
print val, "remainder of two"
else:
print "final case"
final case
2 no remainder
4 no remainder
5 remainder of two
6 no remainder
final case