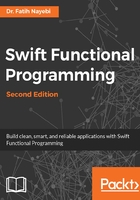
Optionals
Swift provides optionals so they can be used in situations where a value may be absent. An optional will have some or none values. The ? symbol is used to define a variable as optional. Consider the following example:
// Optional value either contains a value or contains nil
var optionalString: String? = "A String literal"
optionalString = nil
The ! symbol can be used to forcefully unwrap the value from an optional. For instance, the following example forcefully unwraps the optionalString variable:
optionalString = "An optional String"
print(optionalString!)
Force unwrapping the optionals may cause errors if the optional does not have a value, so it is not recommended to use this approach as it is very hard to be sure if we are going to have values in optionals in different circumstances. The better approach would be to use the optional binding technique to find out whether an optional contains a value. Consider the following example:
let nilName: String? = nil
if let familyName = nilName {
let greetingfamilyName = "Hello, Mr. \(familyName)"
} else {
// Optional does not have a value
}
Optional chaining is a process to query and call properties, methods, and subscripts on an optional that might currently be nil. Optional chaining in Swift is similar to messaging nil in Objective-C, but in a way that works for any type and can be checked for success or failure. Consider the following example:
class Residence {
var numberOfRooms = 1
}
class Person {
var residence: Residence?
}
let jeanMarc = Person()
// This can be used for calling methods and subscripts through optional chaining too
if let roomCount = jeanMarc.residence?.numberOfRooms {
// Use the roomCount
}
In this example, we were able to access numberOfRooms, which was a property of an optional type (Residence) using optional chaining.
Optionals and optional binding and chaining will be covered in detail in Chapter 7, Dealing with Optionals.