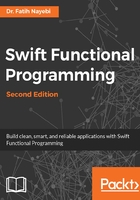
上QQ阅读APP看书,第一时间看更新
Collections
Swift provides typed collections such as array, dictionaries, and sets. In Swift, unlike Objective-C, all elements in a collection will have the same type, and we will not be able to change the type of a collection after defining it.
We can define collections as immutable with let and mutable with var, as shown in the following example:
// Arrays and Dictionaries
var cheeses = ["Brie", "Tete de Moine", "Cambozola", "Camembert"]
cheeses[2] = "Roquefort"
var cheeseWinePairs = [
"Brie":"Chardonnay",
"Camembert":"Champagne",
"Gruyere":"Sauvignon Blanc"
]
cheeseWinePairs ["Cheddar"] = "Cabarnet Sauvignon"
// To create an empty array or dictionary
let emptyArray = [String]()
let emptyDictionary = Dictionary<String, Float>()
cheeses = []
cheeseWinePairs = [:]
The for-in loops can be used to iterate over the items in collections.