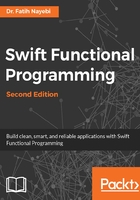
Initialization
The process of preparing an instance of a class, structure, or enumeration for use is called initialization. Classes and structures must set all of their stored properties to an appropriate initial value by the time an instance of that class or structure is created. Stored properties cannot be left in an intermediate state. We can modify the value of a constant property at any point during initialization as long as it is set to a definite value by the time initialization finishes. Swift provides a default initializer for any structure or base class that provides default values for all of its properties and does not provide at least one initializer itself. Consider the following example:
class ShoppingItem {
var name: String?
var quantity = 1
var purchased = false
}
var item = ShoppingItem()
The struct types automatically receive a member-wise initializer if we do not define any of our own custom initializers, even if the struct's stored properties do not have default values.
Swift defines two kinds of initializers for class types:
- Designated initializers: Methods that are able to fully initialize the object
- Convenience initializers: Methods that rely on other methods to complete initialization