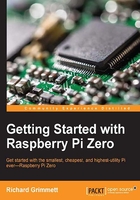
Creating and running Python programs
Now that you are ready to begin programming, you'll need to choose a language. There are many available, such as C, C++, Java, Python, Perl, and a great deal of other possibilities. I'm going to initially introduce you to Python for two reasons: it is a simple language that is intuitive and very easy to use, and it uses a lot of the open source functionality of the robotics world. We'll also cover a bit of C/C++ in this chapter, as some features are only available in C/C++. However, it makes sense to start with Python. To work through the examples in this section, you'll need a version of Python installed. Fortunately, the basic Raspbian system has one already, so you are ready to begin.
We are only going to cover some of the very basic concepts here. If you are new to programming, there are a number of different websites that provide interactive tutorials. If you'd like to practice some of the basic programming concepts in Python using these tutorials, visit https://www.codeacademy.com or http://www.learnpython.org/ or https://docs.python.org and give it a try.
In this section, we'll cover how to create and run a Python file. It turns out that Python can be used interactively, so you can type in the commands one at a time. Using it interactively is extremely helpful when you are getting acquainted with the language features and modules. But you want to use Python to create programs, so you are going to type in your commands using Emacs and then run them in the command line by invoking Python. Let's get started.
Open an example Python file by typing emacs example.py
. Now, let's put some code in the file. Start with the code shown in the following screenshot:

Let's go through the code to see what is happening. The code lines are as follows:
a = input("Input value: ")
: One of the basic purposes of a program is to get input from the user;input
allows us to do that. The data will be input by the user and stored ina
. The promptInput value:
will be shown to the user.b = input("Input second value: ")
: This data will also be input by the user and stored inb
. The promptInput second value:
will be shown to the user.c = a + b
: This is an example of something you can do with the data; in this example, you can adda
andb
.print c
: Another basic purpose of our program is to print out results. Theprint
command prints out the value ofc
.
Note
This code is written using Python 2. If you are using Python 3, you will need to change your print
to print(c)
. For other changes that might be required, go to http://learntocodewith.me/programming/python/python-2-vs-python-3/.
Once you have created your program, save it (using Ctrl + X and Ctrl + S) and quit Emacs (using Ctrl + X and Ctrl + C). Now, from the command line, run your program by typing python example.py
. The result you see should be similar to the following screenshot:

You can also run the program from the command line without typing python example.py
by adding the #!/usr/bin/python
line to the program. Then the program looks similar to the following screenshot:

Adding #!/usr/bin/python
as the first line, simply makes this file available for us to execute from the command line. Once you have saved the file and exited Emacs, type chmod +x example.py
. This will change the file's execution permissions, so the computer will now accept and execute it.
You should be able to simply type ./example.py
and see the program run, as shown in the following screenshot:

Note that if you simply type example.py
, the system will not find the executable file. Here, the file has not been registered with the system, so you have to give the system a path to it. In this case, ./
is the current directory.
Tip
Downloading the example code
You can download the example code files for this book from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
You can download the code files by following these steps:
- Log in or register to our website using your e-mail address and password.
- Hover the mouse pointer on the SUPPORT tab at the top.
- Click on Code Downloads & Errata.
- Enter the name of the book in the Search box.
- Select the book for which you're looking to download the code files.
- Choose from the drop-down menu where you purchased this book from.
- Click on Code Download.
Once the file is downloaded, please make sure that you unzip or extract the folder using the latest version of:
- WinRAR/7-Zip for Windows
- Zipeg/iZip/UnRarX for Mac
- 7-Zip/PeaZip for Linux