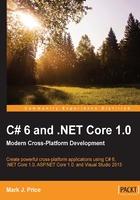
Selection statements
Every application needs to be able to select from choices and branch along different code paths. The two selection statements in C# are if
and switch
, also known as if-else
and switch-case
. You can use if
for all your code but switch
can simplify your code in some common scenarios.
Start Microsoft Visual Studio 2015. In Visual Studio, press Ctrl + Shift + N or choose File | New | Project….
In the New Project dialog, in the Installed Templates list, select Visual C#. In the list at the center, select Console Application, type the name Ch03_SelectionStatements, change the location to C:\Code, type the solution name Chapter03, and then click on OK.
At the top of the Program.cs
file, statically import the System.Console
type, as follows:
using static System.Console;
The if-else statement
The if-else
statement determines which branch to follow by evaluating a Boolean expression. The else
block is optional. if-else
statements can be nested and combined. Each Boolean expression can be independent of the others.
Add the following statements to the Main
method to check whether this console application has any arguments passed to it:
if (args.Length == 0) { WriteLine("There are no arguments."); } else { WriteLine("There is at least one argument."); }
Since there is only a single statement inside each block, this code can be written without the curly braces, as follows:
if (args.Length == 0) WriteLine("There are no arguments."); else WriteLine("There is at least one argument.");
This style of the if-else
statement is not recommended because it can introduce serious bugs, for example, the infamous #gotofail bug in Apple's iPhone operating system. For 18 months after Apple's iOS 6 was released, it had a bug in its Secure Sockets Layer (SSL) encryption code, which meant that any user running Safari to connect to secure websites, such as their bank, for the purpose of payment transactions and other online banking activities, were not properly secure because an important check was being accidently skipped.
Just because you can leave out the curly braces, doesn't mean you should. Your code is not "more efficient" without them, instead, it is less maintainable and potentially more dangerous, as this tweet points out:

The switch-case statement
The switch-case
statement is different from the if-else
statement because it compares a single expression against a list of possible cases. Every case is related to the single expression. Every case must end with the break
keyword (such as case 1 in the following code) or the goto case
keywords, (such as case 2 in the following code) or they should have no statements (such as case 3 in the following code).
Enter the following code after the if-else
statement you wrote previously. Note that the first line is a label that can be jumped to and the second line generates a random number. The switch
statement branches based on the value of this random number:
A_label: var number = (new Random()).Next(1, 7); WriteLine($"My random number is {number}"); switch (number) { case 1: // must be a literal value WriteLine("One"); break; // jumps to end of switch statement case 2: WriteLine("Two"); goto case 1; case 3: case 4: WriteLine("Three or four"); goto case 1; case 5: // go to sleep for half a second System.Threading.Thread.Sleep(500); goto A_label; default: WriteLine("Default"); break; } // end of switch statement
You can use the goto
keyword to jump to another case or a label. The goto
keyword is frowned upon by most programmers but can be the best solution in some scenarios. Use it sparingly.
Run the program by pressing Ctrl + F5. Run it multiple times to see what happens in various cases of random numbers, as shown in the following output:
There are no arguments. My random number is 5 My random number is 3 Three or four One
Autoformatting code
Let's take a persion for a minute to talk about formatting. Code is easier to read and understand if it is consistently indented and spaced out.
If your code can compile, then Visual Studio can automatically format it so it's nicely spaced and indented.
Type the following code (Visual Studio will autoformat even at the end of each line so when it does so, force the code to be improperly spaced out as shown):
var x =3; if(x==3) { WriteLine("three"); }
Press Shift + F6 and wait for your code to build, and then press Ctrl + K, D. Your code will now look like this:
var x = 3; if (x == 3) { WriteLine("three"); }