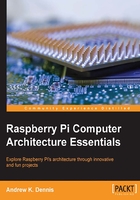
The Python language
Software Engineer Guido van Rossum founded what would come to be known as the Python programming language in the late 1980s. Spawned from a project he was working on to develop an interpreter for a scripting language, he chose the working title Python derived from the popular British comedy series Monty Python's Flying Circus.
The official Python website can be found at https://www.python.org/.
Unlike the C language, Python is an interpreted language. This in essence means that the language's instructions are performed by an interpreter rather than compiled down to machine code.
You can read more about interpreted languages here https://en.wikibooks.org/wiki/Introduction_to_Programming_Languages/Interpreted_Programs.
The most widely used implementation of the Python language is known as CPython, which is written in C. This is also the implementation you will be using on the Raspbian operating system.
You can read more about this implementation at https://docs.python.org/2/c-api/.
This means the Python language is also extensible via C and C++. The Python website provides a guide to extending its functionality at https://docs.python.org/2/extending/extending.html.
The two popular versions of the language are version 2 and version 3.
There are some backward incompatibility issues between version 3 and 2 of the language. Currently, version 2 has wider adoption. In this book we will therefore be using version 2. Version 2 also comes shipped by default with Raspbian.
Let's now take a look at writing a simple application that performs a similar task to that of the C program we just completed.
A simple Python program
We are going to start by opening the IDLE Python development tool. IDLE stands for Integrated DeveLopment Environment and is the program you can use on your Raspberry Pi to directly write Python applications and run them.
At the command line, type the following command:
python
Once loaded you will see a blank document with some information about the version of Python displayed at the top. The following screenshot shows an example:
Example of IDLE on the Raspberry Pi.
In Python, white spacing is very important. Unlike C we do not use braces to signify the start and end of a function, but rather white space indentation.
In our example programs we will use four spaces of indentation.
Our first simple program contains a prompt to the user to enter an integer and then outputs it.
Type the following into IDLE:
def main(): a = raw_input("Please enter an integer: "); print "You entered the number : ", a
When you have finished adding the code, press the Return key twice. You will now see the prompt again.
Into this, type the following command:
main()
You should now be requested for input. Enter a number, for example, 8, and then press Return.
This number should now be displayed with the message You entered the number: prefixing it. This was even easier to write and execute than the Assembly and C program: we needed less code and did not have to run a compiler or linker!
Let's take a look at what each section of the program does.
The first piece of code we entered was the function signature. This has some similarities to the C program we wrote, in that it is called main.
However, unlike the C program, we could have called this anything, for example hello().
If you wish, try re-entering the program with a different name for the function and execute it again. You'll see that it still works.
Following the function definition, we define a variable called a. Python does not force us to define the variable type (for example int) via a prefix when we declare it. We can simply give the variable a name and that will suffice.
The raw_input() function prompts the user and assigns the value typed in by the user to the a variable.
Following this, we output the user's input using the print statement. Therefore, in three lines we have achieved that which took eight in C.
Another interesting property of our program is that unlike our C program we can type any value we want into the prompt, whether it is an integer or not.
You can try this for yourself and see what happens.
While using IDLE is great, if we shut down IDLE our program vanishes. So how do we get around that?
Running a Python program from a file
As we develop projects for our Raspberry Pi we want to be able to save our programs into a file and execute them this way. This allows for re-use. We also may not want to use IDLE but our favorite text editor.
Thankfully we can and shall now walk through the steps to do this.
Create a new directory under your /home/pi/ user called python_programs.
Into this we are going create a new file called first_python_prog.py.
For example, you can use the touch command to create an empty file:
touch first_python_prog.py
Open this file up in your text editor and copy the code in from our earlier example. This will be the code you typed into IDLE.
Once complete at the top of your file you will need to add the shebang:
#!/usr/bin/python
The shebang contains the path to the version of Python being run. This allows the script to be standalone without having to type python before it as you will see shortly.
To the bottom of the file you will also need to add the following:
if __name__ == '__main__': main()
This piece of code checks to see if the Python interpreter has set the __name__ variable to __main__, meaning that the program being run is the main program. If this is true, then it executes the main() function. We did not have to call our function main. Unlike C, Python will allow you to name the entry point to your program anything you like. However, for consistency in our projects we will use the name main.
You can read more about Python runtime services on the official website, where __main__ is also touched upon, at https://docs.python.org/2/library/python.html.
Having added the code, the file should look as follows:
#!/usr/bin/python def main(): a = raw_input("Please enter an integer: "); print "You entered the number: ", a if __name__ == '__main__': main()
Save this code and return to the command line.
To run the application, we can simply type the following command:
python first_python_prog.py
You should now see on the screen a prompt to enter some text. Add this and press return and it will be output back to you.
As with the earlier example of this program, the application will take text characters as well as integers.
We mentioned that the shebang means we do not need to add the command python before running the script. However, before we can simply execute the file from the command line we need to set the permissions on the file to allow it to execute.
In the terminal type this command:
chmod +x first_python_prog.py
Now you can try running the script again by typing the following:
./first_python_prog.py
Once again the prompt should appear.
Tip
You can read more about chmod here: https://en.wikipedia.org/wiki/Chmod
This wraps up our Python section. Here we created the same program in Python as we did in C to compare the complexity and syntax.
We will explore the Python language further in future chapters in which we build a web server.
Let's now review what we have learned so far.