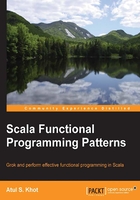
Chapter 2. Singletons, Factories, and Builders
It may sound funny—and I may be stating the obvious—but everyone needs to be born at some point. Go ahead and have a good laugh at that. It is that obvious. Objects, too, need to be born at some point to do useful work. Objects have a lifetime as well. An object is constructed—and hopefully it does something useful before it eventually dies.
In Java we can see the object such as:
Point p = new Point(23, 94);
We know what is going on—an object of class point is created; its constructor-invoked p
is a reference to this newly created object.
At times, we want explicit control of the object-creation process. There are times when we want to allow creation of only one instance of a class. Creational design patterns deal with object-creation mechanisms. Refer to https://sourcemaking.com/design_patterns/creational_patterns for more information on creational patterns.
Creational patterns help create objects in a manner suitable to the situation. Some expensive objects may need to be lazy-initialized. Refer to http://martinfowler.com/bliki/LazyInitialization.html for a very nice introduction to lazy initialization.
Scala provides some nifty ways for lazy initialization, as we will soon see.
We will first look at singletons and then Null Object, a specialized singleton, to avoid null checks. We will use our understanding to implement a set of numbers as a Binary Search Tree (BST). Scala Options are an alternative to null check-based programming. We will rewrite the set implementation in Scala using Options. We will also look at how singletons work in Scala and related idioms.
The next pattern we will look at is factories. We will look at the Java version first and redo the solution in Scala. We will look at some related Scala idioms too.