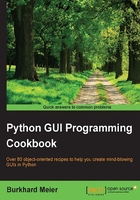
Creating tabbed widgets
In this recipe, we will create tabbed widgets to further organize our expanding GUI written in tkinter.
Getting ready
In order to improve our Python GUI using tabs, we will start at the beginning, using the minimum amount of code necessary. In the following recipes, we will add widgets from previous recipes and place them into this new tabbed layout.
How to do it...
Create a new Python module and place the following code into this module:
import tkinter as tk # imports from tkinter import ttk win = tk.Tk() # Create instance win.title("Python GUI") # Add a title tabControl = ttk.Notebook(win) # Create Tab Control tab1 = ttk.Frame(tabControl) # Create a tab tabControl.add(tab1, text='Tab 1') # Add the tab tabControl.pack(expand=1, fill="both") # Pack to make visible win.mainloop() # Start GUI
This creates the following GUI:

While not amazingly impressive as of yet, this widget adds another very powerful tool to our GUI design toolkit. It comes with its own limitations in the minimalist example above (for example, we cannot reposition the GUI nor does it show the entire GUI title).
While in previous recipes, we used the grid layout manager for simpler GUIs, we can use a simpler layout manager and "pack" is one of them.
In the preceding code, we "pack" tabControl ttk.Notebook into the main GUI form expanding the notebook tabbed control to fill in all sides.

We can add a second tab to our control and click between them.
tab2 = ttk.Frame(tabControl) # Add a second tab tabControl.add(tab2, text='Tab 2') # Make second tab visible win.mainloop() # Start GUI
Now we have two tabs. Click on Tab 2 to give it the focus.

We would really like to see our windows title. So, to do this, we have to add a widget to one of our tabs. The widget has to be wide enough to expand our GUI dynamically to display our window title. We are adding Ole Monty back, together with his children.
monty = ttk.LabelFrame(tab1, text=' Monty Python ') monty.grid(column=0, row=0, padx=8, pady=4) ttk.Label(monty, text="Enter a name:").grid(column=0, row=0, sticky='W')
Now we got our Monty Python inside Tab1.

We can keep placing all the widgets we have created so far into our newly created tab controls.

Now all the widgets reside inside Tab1. Let's move some to Tab2. First, we create a second LabelFrame to be the container of our widgets relocating to Tab2:
monty2 = ttk.LabelFrame(tab2, text=' The Snake ') monty2.grid(column=0, row=0, padx=8, pady=4)
Next, we move the check and radio buttons to Tab2, by specifying the new parent container, which is a new variable we name monty2
. Here is an example which we apply to all controls that relocate to Tab2:
chVarDis = tk.IntVar() check1 = tk.Checkbutton(monty2, text="Disabled", variable=chVarDis, state='disabled')
When we run the code, our GUI now looks different. Tab1 has less widgets than it had before when it contained all of our previously created widgets.

We can now click Tab 2 and see our relocated widgets.

Clicking the relocated Radiobutton(s) no longer has any effect, so we will change their actions to rename the text property, which is the title of the LabelFrame widget, to the name the Radiobuttons display. When we click the Gold Radiobutton, we no longer set the background of the frame to the color gold but here replace the LabelFrame text title instead. Python "The Snake" now becomes "Gold".
# Radiobutton callback function def radCall(): radSel=radVar.get() if radSel == 0: monty2.configure(text='Blue') elif radSel == 1: monty2.configure(text='Gold') elif radSel == 2: monty2.configure(text='Red')
Now, selecting any of the RadioButton widgets results in changing the name of the LabelFrame.

How it works...
After creating a second tab, we moved some of the widgets that originally resided in Tab1 to Tab2. Adding tabs is another excellent way to organize our ever-increasing GUI. This is one very nice way to handle complexity in our GUI design. We can arrange widgets in groups where they naturally belong, and free our users from clutter by using tabs.
Note
In tkinter
, creating tabs is done via the Notebook
widget, which is the tool that allows us to add tabbed controls. The tkinter notebook widget, like so many other widgets, comes with additional properties that we can use and configure. An excellent place to start exploring additional capabilities of the tkinter widgets at our disposal is the official website: https://docs.python.org/3.1/library/tkinter.ttk.html#notebook