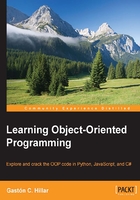
Understanding classes and instances
In the previous chapter, you learned some of the basics of the object-oriented paradigm, including classes and objects, also known as instances. Now, when you pe deep into the programming languages, the class is always going to be the type and the blueprint. The object is the working instance of the class, and one or more variables can hold a reference to an instance.
Let's move to the world of our best friends, the dogs. If we want to model an object-oriented application that has to work with dogs and about a dozen dog breeds, we will definitely have a Dog
abstract class. Each dog breed required in our application will be a subclass of the Dog
superclass. For example, let's assume that we have the following subclasses of Dog
:
TibetanSpaniel
: This is a blueprint for the dogs that belong to the Tibetan Spaniel breedSmoothFoxTerrier
: This is a blueprint for the dogs that belong to the Smooth Fox Terrier breed
So, each dog breed will become a subclass of Dog
and a type in the programming language. Each dog breed is a blueprint that we will be able to use to create instances. Brian
and Merlin
are two dogs. Brian
belongs to the Tibetan Spaniel breed, and Merlin
belongs to the Smooth Fox Terrier breed. In our application, Brian
will be an instance of the TibetanSpaniel
subclass, and Merlin
will be an instance of the SmoothFoxTerrier
subclass.
As both Brian
and Merlin
are dogs, they will share many attributes. Some of these attributes will be initialized by the class, because the dog breed they belong to determines some features, for example, the area of origin, the average size, and the watchdog ability. However, other attributes will be specific to the instance, such as the name, weight, age, and hair color.