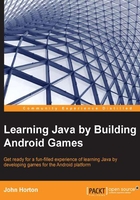
Building and installing our game
Soon, we will actually see our menu in action. But before we do that, we need to find out how to use the Android emulators and how to build our game. Then we will put these together and put our game into an emulator or real device to see it as our players would.
Emulators and devices
Now we have the first part of our game ready to run. We need to test it to check for any errors, crashes, or anything else unintended. It is also important to ensure that it looks good and runs correctly on the device types/sizes and that you want to target.
Note
We will not go into any details about handling different device types. All our games are fullscreen and we will later lock the orientation and dynamically calculate aspects such as screen resolution. So we can get away with writing for a single device type and focus on learning Java.
It might be useful to know for now that you can create a different layout file for any screen size categorization or pixel density. All you need to do is place the layout file using exactly the same filename in the appropriate folder. The Android device will then know the most appropriate layout for it to use. For a detailed discussion, see the Google developers website at http://developer.android.com/guide/practices/screens_support.html.
Note that you do not need to understand any of the information at the preceding link to learn Java and publish your first games.
There are a few options to do this and we will look at two. First, we will use Android Studio and the Android Development Tools to make a device emulator so that we can use, test, and debug our games on a wide range of device emulators on the same PC/Mac we are developing on. So we don't need to own a device. This will allow us to get crash reports from our games.
Then we will install the game directly to a real device so that we can see exactly what the owner of that device will see when they download our app.
There are more options. For example, you can connect a real device via USB and debug directly on the device with the errors and syntactical feedback in Android Studio. The process for this might vary for different devices and since we won't be focusing on anything but basic debugging, we will not cover that in this book.
Let's get our emulator up and emulating:
- On the right-hand side of the Android Studio quick launch bar, find the AVD manager icon:
- Click on the icon to start the Android Virtual Device Manager. Then click on the Create Virtual Device... button on the bottom-left side to bring up the Virtual Device Configuration window.
- Now click on the Nexus 4 option and then click on Next.
- Now we need to choose the version of Android we will use to build and test our games on. The latest version (at time of writing) is Lollipop - 21 - x86. It is the only option where we don't need to complete a download to continue. So select it (or whatever the default is at the time you are reading this) and then click on Next to continue.
- On the next screen we can leave all the default settings. So click on Finish.
Now we will launch (switch on) our virtual device then actually run our game that we made earlier by performing the following steps:
- Click on Nexus 4 API 21 under the Name column. Now click on the triangular play icon to the right of the description of our emulator.
- Once it has started, unlock the device by clicking and dragging anywhere on the screen of the emulated device. This is analogous to swiping to unlock a real Nexus 4. Here is what our Nexus 4 virtual device looks like when it is running and unlocked:
You can play with this emulator in almost the same way as you can a real Android device. However, you cannot download apps from Google Play. You might notice that the emulator is a bit slow compared to a real device, even compared to an old one. Shortly, we will look at running our apps on a real device.
Once the emulator is running, it's usually best to leave it running so that each time we want to use it, we don't have to wait for it to start. Let's use the emulator:
- Launch the emulator if it is not already running and make sure the device is unlocked as described previously.
- Click on the run icon in the toolbar (shown next) to run your app. You can achieve the same thing by navigating to Run | Math Game Chapter 2 from the menu bar:
- After a pause while Android Studio builds our application, a pop-up dialog will ask you which device you want to run the app on. Choose the device with Nexus 4 API 21 in the description. This is the already running device that we created earlier. Now press OK.
- Notice at this point that the useful Android window appears at the bottom section of Android Studio. In the unlikely event of you having any problems, just check for typos in the code. If things really don't work out, just go back to the Using the sample code section to compare with or copy and paste the supplied code.
After another pause, our game menu screen will appear on the emulator. Of course, it doesn't do anything yet, but it is running and the buttons can be pressed.
When you're done, you can press the back or home icons to quit the application, just as you would on a real Android device.
Now we have seen one of the ways we can test our app by running it in the Android emulator. Let's find out how to make our code into an app we can distribute and use on a real device.
Building our game
To run our game on a real Android device, we need to create a .apk
file, that is, a file that ends with the extension .apk
. A .apk
file is a compressed archive of files and folders that the Android system uses to run and install our app. These are the steps to use Android Studio to make a .apk
of our game:
- From the menu bar, navigate to Build | Generate Signed APK.
- A slightly verbose window will pop up and say: For Gradle-based projects, the signing configuration should be specified in the Gradle build scripts. You can safely dismiss this window by clicking on OK.
- Next up is the Generate Signed APK Wizard dialog. Here, we are creating a key that identifies the key holder as authorized to distribute the APK. At the end of this process, you will have a
.keys
file that you can use each time you build a.apk
file. So this step can be missed out in future. Click on the Create new button. - In the Key Store Path field, type or go to a location on your hard drive where you would like to store your key. You will then be prompted to choose a filename for the keystore. This is arbitrary. Type
MyKeystore
and click on OK. - Type a password in the Password field and then retype it in the Confirm field. This is the password to a store that will help protect your key.
- Next, in the Alias field, type a memorable alias. You can think of this as a kind of username for your key. Again type a password in the Password field and then retype it in the Confirm field. This is the password to your key.
- Leave the Validity Years dropdown at the default of 25.
- You can then fill out your Name and organization details (if any) and click on OK.
- Now our key and keystore are complete, and we can click on OK on the Generate Signed APK wizard dialog.
- We are then prompted to select Run Proguard. Encrypting and optimizing our
.apk
is unnecessary at this time. So just click on Finish to generate our app's.apk
file. - The generated
.apk
file will be put in the same directory that you chose to put the project files. For example,MathGameChapter2/app
.
We have now built a .apk
file that can be run on any Android device that was specified when we first created the project.
Installing the setup to a device
So we have our .apk
file and we know were to find it. Here is how we will run it on our Android device.
We can use one of a number of methods to get the .apk
file into the device. The method I find one of the easiest is the use of a cloud storage service such as Dropbox. You can then simply click and drag the .apk
file to your Dropbox folder and you're done. Alternatively, your Android device probably came with PC synchronization software that allows you to drag and drop files to and from your device. After you have placed the .apk
file on your Android device, continue with the tutorial.
Most Android phones are set not to install apps from anywhere except the Google Play Store. So we need to change this. The exact menus you will navigate to might vary very slightly on your device but the following options tend to be almost the same on most devices, old and new:
- Find and tap the Settings app. Most Android phones also have a Settings menu option. Either will do. Now select Security and scroll down to the Unknown sources option. Tap the Unknown sources checkbox to allow apps to be installed from unknown sources.
- Locate the file on your Android device using the Dropbox app or your devices file browser depending on the method you chose to put the APK on your device. Tap the
MathGameChapter2.apk
file. - You can now install the app just like any other. When prompted, press Install and then Open. The game will now be running on your device.
Hold your device in a portrait orientation as this is how the UI was designed. Congratulations on running your very own Android app on your own device. In a later version of the math game, we will lock the orientation to make this more user friendly.
Future projects
Throughout the book, we will test and run our game projects. It is entirely up to you which of the methods we discussed you prefer. If you are getting crashes or unexplained bugs, then you will need to use an emulator. If all is working well, then the quickest and probably most pleasing way will be to run it on a device you own.