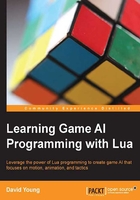
上QQ阅读APP看书,第一时间看更新
Avoiding blocks and agents
Modifying the SeekingAgent.lua
script by adding the weighted sum of the ForceToAvoidAgents
and ForceToAvoidObjects
functions allows for the seeking agent to avoid potential collisions. When running the sandbox, try shooting boxes in the path of the agent and watch it navigate around the boxes:
SeekingAgent.lua
function Agent_Update(agent, deltaTimeInMillis) local destination = agent:GetTarget(); local deltaTimeInSeconds = deltaTimeInMillis / 1000; local avoidAgentForce = agent:ForceToAvoidAgents(1.5); local avoidObjectForce = agent:ForceToAvoidObjects(1.5); local seekForce = agent:ForceToPosition(destination); local targetRadius = agent:GetTargetRadius(); local radius = agent:GetRadius(); local position = agent:GetPosition(); local avoidanceMultiplier = 3; -- Sum all forces and apply higher priority to avoidance -- forces. local steeringForces = seekForce + avoidAgentForce * avoidanceMultiplier + avoidObjectForce * avoidanceMultiplier; AgentUtilities_ApplyForce( agent, steeringForces, deltaTimeInSeconds); ... end
Sandbox.lua
:
require "DebugUtilities"; function Sandbox_Update(sandbox, deltaTimeInMillis) -- Grab all Sandbox objects, not including agents. local objects = Sandbox.GetObjects(sandbox); -- Draw debug bounding sphere representations for objects with -- mass. DebugUtilities_DrawDynamicBoundingSpheres(objects); end
Now when we run the sandbox, we can shoot boxes at our seeking agent and watch it steer around each object.

Avoiding obstacles using avoidance