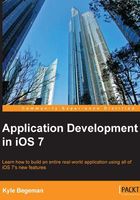
Modules
While developing applications using Xcode and the iOS SDK, you may have noticed that it has never been a requirement to import commonly used header files, such as UIViewController.h
or UIView.h
.
Open any file in any project, and navigate to any view-controller based .h
file in the project. The very first line of code will read as follows:
#import <UIKit/UIKit.h>
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
As an iOS developer, you have probably written hundreds of #import
statements in any one project. When the compiler reaches an import statement, it literally inserts every line of code found in the imported header file. In the previous example of the first line of code, UIKit.h
imports all header files available in the UIKit Framework; so, you don't have to worry about which header file should be imported for different instances.
If you have ever taken a look at all of the files included in UIKit, you will see that they total over 11,000 lines of code. This means that each file importing UIKit.h
will grow by 11,000 lines of code. This is less than ideal; however, Apple provides one solution with precompiled header (PCH) files.
Precompiled headers – a partial solution
Each project you create will automatically generate its own PCH file in the supporting files group. During the preprocessing phase of compilation, the PCH file will load and cache the specified headers to import. The following is an example of a PCH file:
#import <Availability.h> #ifndef __IPHONE_5_0 #warning "This project uses features only available in iOS SDK 5.0 and later." #endif #ifdef __OBJC__ #import <UIKit/UIKit.h> #import <Foundation/Foundation.h> #import "UIImage+ImageEffects.h" #endif
Your application may require a specific framework or class in multiple files. Rather than importing the file individually (and repeatedly), adding the import statement to the PCH file will precompute and cache a majority of the work during the preprocessing phase of compilation. This allows each file to be pulled from the cache when available.
Although this method works well, when importing the Apple frameworks, you must always remember to link the frameworks to your project. Failing to do so will result in a number of errors thrown by the compiler.
Modules – smart importing
With the introduction of iOS 7, Apple has introduced a new way to handle precompiling frameworks with modules. Instead of replacing an import statement with every line of code, a module encapsulates a framework into a self-contained block. Modules are precompiled in the same way import statements are precompiled in the PCH file; however, using modules will automatically link the proper framework and provide the exact same speed boost to compilation.
Modules are enabled by default in all new projects created using Xcode 5. For older projects, you can enable modules in your project's build settings by searching for modules and setting Enable Modules (C and Objective-C) to Yes.

Now that modules have been turned on, you can start using the new syntax to import frameworks. At the top of the .h
file you wish to import, simply type the following code:
@import QuartzCore;
That's all that is required in your code. Xcode will automatically link the required framework (in this case, QuartzCore
) and provide you with all of the speed boosts for compilation.
Additionally, you can import specific header files based on need. You may, for instance, only require the CoreAnimation
headers provided by QuartzCore
. You can easily import these headers by typing the following:
@import QuartzCore.CoreAnimation;
Additionally, Xcode will automatically convert #import
statements to @import
for you at runtime. Although convenient, it is still recommended you update to new syntax whenever possible.
It is also important to note that modules currently only support Apple frameworks. Custom classes and third-party frameworks still require the traditional method or the PCH file.