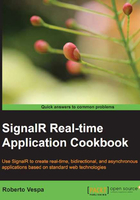
Removing a connection from a group
As we just saw, groups are an interesting and useful feature exposed by the Hub API. In the previous recipe, we concentrated on how to add connections to a specific group, but we did not expand on how you can remove them. This will be the subject of this recipe.
It's quite obvious that in order to remove connections from a group, we'll have to add them to it first; however, that part has already been covered by the previous recipe. Here we'll show the code to do both operations in order to deliver a fully working recipe, but we'll indulge in comments only for the removal part. For more details about adding connections to a group, please refer to the previous recipe.
Getting ready
Before proceeding, we create a new empty web application, which we'll call Recipe09
.
How to do it…
We're ready to actually start adding our SignalR bits by performing the following steps:
- We need a Hub called
EchoHub
. - We then need an OWIN Startup class named
Startup
containing just a simpleapp.MapSignalR();
call inside theConfiguration()
method to bootstrap SignalR. - We finally need to edit the content of the Hub with the following code:
using Microsoft.AspNet.SignalR; using Microsoft.AspNet.SignalR.Hubs; namespace Recipe09 { [HubName("echo")] public class EchoHub : Hub { public void Subscribe(string groupName) { Groups.Add(Context.ConnectionId, groupName); } public void Unsubscribe(string groupName) { Groups.Remove(Context.ConnectionId, groupName); } public void Hello(string groupName) { var msg = string.Format("Welcome from {0}", groupName); Clients.Group(groupName).greetings(msg); } } }
What's important here? We need to make sure that we perform the following steps:
- The
Hello()
andSubscribe()
methods, although important, are the same as we saw in the previous recipe, so we skip the details about them - The
Unsubscribe()
method receives agroupName
parameter, and then uses theGroups
member inherited fromHub
to remove theConnectionId
of the caller from the specified group, via theRemove()
method
Basically, we are just adding the removal feature to the Hub from the Broadcasting to all clients in a group except the caller recipe. Let's now create an HTML client page that we'll call index.html
and add the following code to it:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Recipe09</title> <script src="Scripts/jquery-2.1.0.js"></script> <script src="Scripts/jquery.signalR-2.0.2.js"></script> <script src="/signalr/hubs"></script> <script> $(function () { var hub = $.connection.echo; hub.client.greetings = function (message) { var li = $('<li/>').html(message); $('#messages').append(li); }; $.connection.hub .start() .done(function () { var group; $('#subscribe').click(function () { toggler(); group = $('#group').val(); hub.server.subscribe(group); }); $('#unsubscribe').click(function () { toggler(); hub.server.unsubscribe(group); }); $('#send').click(function () { hub.server.hello(group); }); }); var toggler = function () { $('#subscribe').toggle(); $('#unsubscribe').toggle(); $('#send').toggle(); }; }); </script> </head> <body> <label>Group:</label> <select id="group"> <option value="A">A</option> <option value="B">B</option> <option value="C">C</option> </select> <button id="subscribe">Subscribe</button> <button id="unsubscribe" style="display: none">Unsubscribe</button> <button id="send" style="display: none">Send</button> <ul id="messages"></ul> </body> </html>
The preceding code again is pretty similar to the one in the Adding a connection to a group recipe, apart from some jQuery tricks to enable/disable buttons. The only significant addition is the call to the unsubscribe()
method exposed by the server-side Hub, plus the fact that the selected group name is stored in a variable that is to be passed on subsequent subscribe()
/unsubscribe()
calls. Those are not SignalR-specific additions, and their goal should be easy to grasp.
Now we can open index.html
multiple times in different browser windows (or tabs), and then we can click on the Subscribe button after selecting one of the three available groups, or the Unsubscribe button when subscribed. When subscribed, we can click on the Say Hello! button on any of the browser windows that we have and see messages going around; when unsubscribed, we won't be able to send anything, and we won't see any message popping up.