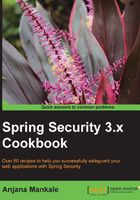
Form-based authentication on servlet
In the previous sections, we demonstrated the basic authentication on servlets and JSPs. Now let's use form-based authentication on servlets.
Getting ready
Let's apply form-based authentication on servlet. You will need a simple web application with a servlet, a web container to handle the authentication, and the web.xml
file that tells the container what to authenticate.
How to do it...
Let's see some simple steps for implementing form-based authentication on servlets:
- Create a JSP file named
Containerform.jsp
:<%@ page language="java" contentType="text/html; charset=ISO-8859-1" pageEncoding="ISO-8859-1"%> <!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd"> <html> <head> <meta http-equiv="Content-Type" content="text/html; charset=ISO-8859-1"> <title>Insert title here</title> </head> <body> <form method="POST" action="j_security_check"> Username:<input type="text" name="j_username"> password:<input type="password" name="j_password"> <input type=submit> </form> </body> </html>
What do you observe in the previous code?
action=j_security_check
is the default URL, which is recognized by the web container. It tells the container that it has the user credentials to be authenticated. - Now, edit the
web.xml
file:<login-config> <auth-method>FORM</auth-method> <form-login-config> <form-login-page>/Containerform.jsp</form-login-page> <form-error-page>/logoff.jsp</form-error-page> </form-login-config> </login-config>
Build the project and export the .war
files to JBoss.
How it works...
The previous example demonstrated the Form-based authentication. The J2EE container reads the web.xml
file, the <auth-method>
tag has the form
attribute set. Then it further looks for the login.jsp
file, which needs to be displayed to do form-based authentication. The <form-error-page>
and <form-login-page>
has the login file name and the error page that needs to be displayed on authentication failure. When the user tries to access the secured resource, the J2EE container redirects the request to the login page. The user credentials are submitted to j_security_check
action. This action is identified by the container and does the authentication and authorization; on success the user is redirected to the secured resource and on failure the error page shows up.
The following are the screenshots of the workflow which shows the login page for the user and displays the user information on successful authentication:


See also
- The Form-based authentication with open LDAP and servlet recipe
- The Hashing/Digest Authentication on servlet recipe
- The Basic authentication for JAX-WS and JAX-RS recipe
- The Enabling and disabling the file listing recipe