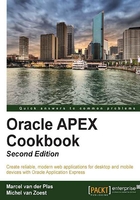
Creating visual effects with JavaScript libraries>
JavaScript libraries can be used to make your web page catchy. You can use JavaScript libraries to pop up or disappear items. But JavaScript libraries can also be used when you want to implement the drag-and-drop functionality.
There are several JavaScript libraries. The most well-known JavaScript library is jQuery. In Apex 4.0, jQuery is built-in and can be directly accessed, so you don't have to download and install it. However, when you want to use a JavaScript library such as scriptaculous, you have to download and install it.
Since jQuery is built-in, we will use it to demonstrate the making of an accordion. We will create an accordion where we put in some information, and by clicking on it, you can see the different sections.
Getting ready
We will use the APP_EVENTS
table, so make sure this table is accessible.
How to do it...
First we create a region template:
- Navigate to Shared Components | Templates.
- Click on the Create button.
- Select Region and click on Next.
- Select From Scratch and click on Next.
- Enter a name for the region, for example
accordion
. - In the Template Class listbox, select Custom 1.
- Click on the Create button.
- In the list of templates, click the accordion template.
- In the Definition section, enter the following in the Template text field:
<div id="#REGION_STATIC_ID#" #REGION_ATTRIBUTES#> #BODY##SUB_REGION_HEADERS##SUB_REGIONS# </div> <link rel="stylesheet" href="#IMAGE_PREFIX#libraries/jquery-ui/1.8/themes/base/jquery.ui.accordion.css" type="text/css" /> <script src="#IMAGE_PREFIX#libraries/jquery-ui/1.8/ui/minified/jquery.ui.accordion.min.js" type="text/javascript"></script> <script type="text/javascript"> apex.jQuery(function() { apex.jQuery("##REGION_STATIC_ID#").accordion(); }); </script> [9672_03_26.txt]
- In the Sub Regions section, enter the following code in the Template text field:
<h3><a href="#">#SUB_REGION_TITLE#</a></h3> <div>#SUB_REGION#</div> [9672_03_27.txt]
- Click on the Apply Changes button.
The template is ready now. The next step is to create a region and a sub-region:
- Go to the page where you want the accordion to appear.
- In the Regions section, click on the Add icon.
- Select HTML and click on Next.
- Again select HTML and click on Next.
- Enter a title for the region, for example
accordion
. - From the Region template listbox, select accordion (the template we just created).
- Click on Next.
- Click on the Create Region button.
That was the region. Now we will create the sub-region:
- In the Regions section, click on the Add icon.
- Select HTML and click on Next.
- Again select HTML and click on Next.
- Enter a title for the sub-region, for example
Welcome
. - From the Region Template listbox, select No Template.
- From the Parent Region listbox, select the parent region we just created, that is, accordion.
- Click on Next.
- In the HTML Region Source, enter some text.
- Click on the Create Region button.
That was the first slice. Now we will create a second slice:
- In the Regions section, click on the Add icon.
- Select Report and click on Next.
- Select SQL Report and click on Next.
- In the Title text field, enter Events.
- In the Region Template listbox, select No Template.
- In the Parent Region listbox, select the parent region we created, that is accordion.
- Click on Next.
- In the Query textarea, enter the following query:
select event , location from app_events; [9672_03_28.txt]
- Click on the Create Region button.
- The accordion is ready now. Run the page and see how it looks. Mine looks something as shown in the following screenshot:
How it works...
This recipe consists of two parts: creating a template of type region and creating regions and sub-regions. The region is the placeholder for the accordion and the sub-regions are the slices. In the template we put some HTML and JavaScript code that is needed to reference the jQuery containing the code for the accordion, and to call the accordion
function.
In the template, the div gets the ID from the #REGION_STATIC_ID#
substitution variable. The accordion
function uses that ID to render the accordion on the proper region. The jQuery call to the accordion includes the div ID, prefixed by the hash sign (#). That is why you see two hash signs in the call to the accordion:
apex.jQuery("##REGION_STATIC_ID#").accordion();
The first is the hash prefix and the second is the enclosing hash sign of the substitution variable.
The displayed data has to conform to the following layout:
<div id="accordion"> <h3><a href="#">seminar</a></h3> <div>30-MAR-2010: Apex day, Zeist, Netherlands</div> <h3><a href="#">workshop</a></h3> <div>07-JUN-2010: APEX 4.0 workshop</div> </div>
The first and the last line are part of the parent region. Everything in between is part of the sub-region (the slices). In the preceding example, you see two slices. Since the <h3>
, <a>
, and the <div>
tags are in the template, you only have to put the text into the sub-region to display as desired.
There's more...
You can make the accordion dynamic by using a PL/SQL dynamic content region where you use sys.htp.p
to display the data including the <h3>
, <a>
, and <div>
tags:
- In the Regions section, click the Add icon.
- Select PL/SQL dynamic content.
- Enter a title for the region, for example
Events
. - In the Region Template listbox, select the accordion template.
- Click on Next.
- In the PL/SQL Source textarea, enter the following code:
declare cursor c_aet is select rownum line , event_type , event_date , event , location from (select decode(row_number() over (partition by event_type order by event_type),1,event_type,null) event_type , event_date , event , location from app_events); begin for r_aet in c_aet loop if r_aet.event_type is not null then if r_aet.line != 1 then sys.htp.p('</div>'); end if; sys.htp.p('<h3><a href="#">'||r_aet.event_type||'</a></h3>'); sys.htp.p('<div>'); end if; sys.htp.p('<b>'||to_char(r_aet.event_date,'DD-MM-YYYY')||'</b>'||': '||r_aet.event||', '||r_aet.location); sys.htp.p('<br>'); end loop; end; [9672_03_29.txt]
This code selects from the APP_EVENTS
table and displays the date and the event. Therefore it uses sys.htp.p
to display the data, including the necessary tags. By using the accordion
template, everything will be displayed in an accordion.
You can select the slices by clicking on them. However, you can change the call to the accordion so that you only have to hover over the slices instead of clicking on them. In the accordion
template, change the following line of code:
apex.jQuery("##REGION_STATIC_ID#").accordion();
Into:
apex.jQuery("##REGION_STATIC_ID#").accordion({event:"mouseover"});
Besides the accordion, jQuery offers other cool "widgets", such as a slider or a progressbar. For more information, take a look at http://www.jquery.com.