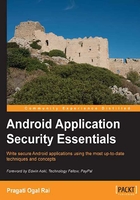
Permission protection levels
At the application level, Android security is based on permissions. Using this permission-based model, the Android system protects system resources, such as camera and Bluetooth, and application resources, such as files and components. An application should have privileges to act upon or use these resources. Any application intending to use these resources needs to declare to the user that it will be accessing these resources. For example, if an application will be sending and reading a SMS, it will need to declare android.permission.SEND_SMS
and android.permission.READ_SMS
in the manifest file.
Permissions are also an effective method for access control between applications. An application's manifest file contains a list of permissions. Any external application that wishes to access this application's resources should possess these permissions. This is discussed in greater detail in the next chapter.
All Android permissions are declared as constants in the Manifest.permission
class. However, this class does not mention the type of permission. This can be used to check the Android source code. I have tried to list some of these permissions in the following sections. The list of permissions keeps changing based on the functionality, so it is best to refer to the Android source code for an up-to-date listing of permissions. For example, android.permission.BLUETOOTH
has been around since API level 1 but android.permission.AUTHENTICATE_ACCOUNTS
was added in API 5. You can get information to get the Android source code at source.android.com.
All Android permissions lie in one of the four protection levels. Permissions of any protection levels need to be declared in the manifest file. Third party apps can only use permissions with protection level 0 and 1. These protection levels are discussed as follows:
- Normal permissions: Permissions in this level (level 0) cannot do much harm to the user. They generally do not cost users money, but they might cause users some annoyance. When downloading an app, these permissions can be viewed by clicking on the See All arrow. These permissions are automatically granted to the app. For example, permissions
android.permission.GET_PACKAGE_SIZE
andandroid.permission.FLASHLIGHT
lets the application get the size of any package and access a flashlight respectively.The following is a list of some of the normal permissions that exist in the Android system at the time of writing the book.
Permissions that are used to set user preferences include:
android.permission.EXPAND_STATUS_BAR
android.permission.KILL_BACKGROUND_PROCESSES
android.permission.SET_WALLPAPER
android.permission.SET_WALLPAPER_HINTS
android.permission.VIBRATE
android.permission.DISABLE_KEYGUARD
android.permission.FLASHLIGHT
Permissions that allow user to access system or application information include:
android.permission.ACCESS_LOCATION_EXTRA_COMMANDS
android.permission.ACCESS_NETWORK_STATE
android.permission.ACCESS_WIFI_STATE
android.permission.BATTERY_STATS
android.permission.GET_ACCOUNTS
android.permission.GET_PACKAGE_SIZE
android.permission.READ_SYNC_SETTINGS
android.permission.READ_SYNC_STATS
android.permission.RECEIVE_BOOT_COMPLETED
android.permission.SUBSCRIBED_FEEDS_READ
android.permission.WRITE_USER_DICTIONARY
Permissions that users should be asked for carefully include
android.permission.BROADCAST_STICKY
, that lets an application send a sticky broadcast which stays alive even after the broadcast has been delivered. - Dangerous permissions: Permissions in this protection level (level 1) are always shown to the user. Granting dangerous permissions to apps allow them to access device features and data. These permissions cause user privacy or financial loss. For example, granting dangerous permissions, such as
android.permission.ACCESS_FINE_LOCATION
andandroid.permission.ACCESS_COARSE_LOCATION
, lets an app access the user's location that might be a privacy concern if the app does not need such a capability. Similarly, granting an appandroid.permission.READ_SMS
andandroid.permission.SEND_SMS
permission lets an application send and receive SMS which might be a privacy issue.At any given point, a user can check the permissions granted to any application by going to the settings and selecting the application. Refer to the following two images that show the permissions for the Gmail application. The first image shows the dangerous permissions that are always displayed to the user. Notice the drop-down menu button Show All. This option shows all the permissions requested by the application. Notice the permission, Hardware controls, and a normal permission that is not displayed to the user by default.
The following is a list of some of the dangerous permissions in the Android system at the time of writing the book.
Some dangerous permissions can be costly for the users. For example, an application that sends a SMS or subscribes to paid feeds can cause users huge bucks. The following are some other examples:
android.permission.RECEIVE_MMS
android.permission.RECEIVE_SMS
android.permission.SEND_SMS
android.permission.SUBSCRIBED_FEEDS_WRITE
Permissions that have the power to change the state of the phone include the following. These should be used carefully, as they can make the system unstable, cause annoyance, and can also make the system less secure. For example, permission to mount and unmount filesystems can change the state of the phone. Any malicious application with the permission to record audio can easily eat up phone's memory with garbage audio. The following are some examples:
android.permission.MODIFY_AUDIO_SETTINGS
android.permission.MODIFY_PHONE_STATE
android.permission.MOUNT_FORMAT_FILESYSTEMS
android.permission.WAKE_LOCK
android.permission.WRITE_APN_SETTINGS
android.permission.WRITE_CALENDAR
android.permission.WRITE_CONTACTS
android.permission.WRITE_EXTERNAL_STORAGE
android.permission.WRITE_OWNER_DATA
android.permission.WRITE_SETTINGS
android.permission.WRITE_SMS
android.permission.SET_ALWAYS_FINISH
android.permission.SET_ANIMATION_SCALE
android.permission.SET_DEBUG_APP
android.permission.SET_PROCESS_LIMIT
android.permission.SET_TIME_ZONE
android.permission.SIGNAL_PERSISTENT_PROCESSES
android.permission.SYSTEM_ALERT_WINDOW
Some dangerous permissions can be privacy risks. Permissions that let users read SMS, logs, and calendar can be easily used by botnets and Trojans to do interesting stuff on a command of their remote owners. The following are some other examples:
android.permission.MANAGE_ACCOUNTS
android.permission.MODIFY_AUDIO_SETTINGS
android.permission.MODIFY_PHONE_STATE
android.permission.MOUNT_FORMAT_FILESYSTEMS
android.permission.MOUNT_UNMOUNT_FILESYSTEMS
android.permission.PERSISTENT_ACTIVITY
android.permission.PROCESS_OUTGOING_CALLS
android.permission.READ_CALENDAR
android.permission.READ_CONTACTS
android.permission.READ_LOGS
android.permission.READ_OWNER_DATA
android.permission.READ_PHONE_STATE
android.permission.READ_SMS
android.permission.READ_USER_DICTIONARY
android.permission.USE_CREDENTIALS
- Signature permissions: Permissions in this protection level (level 2) allow two applications authored by the same developer, access each other's components. This permission is automatically granted to the app if the app being downloaded has the same certificate as the application that declared the permission. For example, application A defines a permission
com.example.permission.ACCESS_BOOK_STORE
. Application B, signed by the same certificate as application A, declares it in its manifest file. Since both application A and B have the same certificate, this permission is not shown to the user when installing the application B. A user can certainly view it, using See All. An app can perform really powerful actions with this permission of this group. For example, withandroid.permission.INJECT_EVENTS
, an app can inject events such as keys, touch, and trackball into any application andandroid.permission.BROADCAST_SMS
can broadcast an SMS acknowledgement. This permission defined by the Android systems that lie in this protection group is reserved for system apps only.Some permissions in this level allow applications to use system level features. For example,
ACCOUNT_MANAGER
permission lets the applications use account authenticators and BRIK permissions allow the applications to brick the phone. The following is a list of some of the signature permissions at the time of writing the book. For a complete reference check the Android source code or theManifest.permission
class:android.permission.ACCESS_SURFACE_FLINGER
android.permission.ACCOUNT_MANAGER
android.permission.BRICK
android.permission.BIND_INPUT_METHOD
android.permission.SHUTDOWN
android.permission.SET_ACTIVITY_WATCHER
android.permission.SET_ORIENTATION
android.permission.HARDWARE_TEST
android.permission.UPDATE_DEVICE_STATS
android.permission.CLEAR_APP_USER_DATA
android.permission.COPY_PROTECTED_DATA
android.permission.CHANGE_COMPONENT_ENABLED_STATE
android.permission.FORCE_BACK
android.permission.INJECT_EVENTS
android.permission.INTERNAL_SYSTEM_WINDOW
android.permission.MANAGE_APP_TOKENS
Some permissions in this level allow applications to send system level broadcasts and intents such as broadcasting intents and SMS. These permissions include:
android.permission.BROADCAST_PACKAGE_REMOVED
android.permission.BROADCAST_SMS
android.permission.BROADCAST_WAP_PUSH
Other permissions in this level allow applications to access system level data that third party applications do not have. These permissions include:
android.permission.PACKAGE_USAGE_STATS
android.permission.CHANGE_BACKGROUND_DATA_SETTING
android.permission.BIND_DEVICE_ADMIN
android.permission.READ_FRAME_BUFFER
android.permission.DEVICE_POWER
android.permission.DIAGNOSTIC
android.permission.FACTORY_TEST
android.permission.FORCE_STOP_PACKAGES
android.permission.GLOBAL_SEARCH_CONTROL
- SignatureOrSystem permissions: As with signature protection level, this permission is granted to applications with the same certificate as the application that defined the permission. In addition, this protection level includes applications with the same certificate as the Android system image. This permission level is mainly used for applications that are built by handset manufacturers, carriers, and system applications. These permissions are not allowed for third party apps. These permissions let apps perform some very powerful functions. For example, the permission
android.permission.REBOOT
allows an app to reboot the device. The permissionandroid.permission.SET_TIME
lets an app set system time.A list of some of the SignatureOrSystem permissions as of the time of writing the book is as follows:
android.permission.ACCESS_CHECKIN_PROPERTIES
android.permission.BACKUP
android.permission.BIND_APPWIDGET
android.permission.BIND_WALLPAPER
android.permission.CALL_PRIVILEGED
android.permission.CONTROL_LOCATION_UPDATES
android.permission.DELETE_CACHE_FILES
android.permission.DELETE_PACKAGES
android.permission.GLOBAL_SEARCH
android.permission.INSTALL_LOCATION_PROVIDER
android.permission.INSTALL_PACKAGES
android.permission.MASTER_CLEAR
android.permission.REBOOT
android.permission.SET_TIME
android.permission.STATUS_BAR
android.permission.WRITE_GSERVICES
android.permission.WRITE_SECURE_SETTINGS