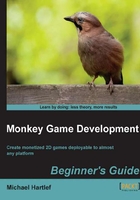
上QQ阅读APP看书,第一时间看更新
Time for action — moving the ball
Moving the ball is as simple as moving the paddles, as you will see.
- For updating the ball's position, you need to create a new method called
UpdateBall
. At first, we will update the ball's X and Y position:Method UpdateBall:Int() bX += bdX 'Add the X speed of the ball to its X position bY += bdY 'Add the Y speed of the ball to its Y position
We could end here, but then the ball would not bounce of the walls and would just disappear in nowhere land.
- Add a check if the ball hits the top wall and reacts to it.
If bY < 10.0 then bY = 10.0 'Set the Y position back to 10.0 bdY *= -1 'Inverse the balls Y speed Endif
- Next, check if the ball hits the bottom wall and, again, reacts to it.
If bY > 470.0 then bY = 470.0 'Set the Y position back to 470.0 bdY *= -1 'Inverse the balls Y speed Endif
- Now, check against the left wall. If it hits it, add a point to the player's points.
If bX < 5.0 then bX = 5.0 'Set the X position back to 5.0 bdX *= -1 'Inverse the balls X speed pPoints += 1 'Add 1 to the player's points
- As a score was made, check if the victory conditions of 10 points are reached. If yes, set the
gameMode
toGameOver
. After that, close theIf
statement.If pPoints >= 10 then gameMode = 2 Print (ePoints + ":" + pPoints) Endif
- The last thing in this method will be the check against the right wall. Again, if it hits, add a point to the enemy's points.
If bX > 635.0 then bX = 635.0 'Set the X position back to 635.0 bdX *= -1 'Inverse the balls X speed ePoints += 1 'Add 1 to the enemies points
- The enemy made a score, so check if the victory conditions of 10 points have been reached. If yes, set the
gameMode
toGameOver
. After that, close theIf
statement. And close the method.If ePoints >= 10 then gameMode = 2 Print (ePoints + ":" + pPoints) Endif End
- This was one of our biggest methods so far. All we need to do now is to add a call to
UpdateBall
into theUpdateGame
method.ControlEnemies() 'Control the enemy UpdateBall() 'Update the ball's position Return True
- Phew! We are getting there. Save your game now and test it. The ball should bounce off the walls now, and if it hits the left or right wall, you should see a message with the current score printed.

If you need a pre-made source file for the next steps, Pongo_07.Monkey
can help you there.