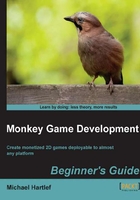
Time for action — implementing some rockets
Surely you know what you have to do now. Like all the other main objects we have created so far, each rocket will have its own class, which is located in its own file.
- Create a new file and save it under the name
rocketClass.monkey
. - Start the script with the usual mumbo jumbo:
Strict
mode, importinggameClasses
, and a globalrockets
list definition.Strict Import gameClasses Global rockets := New List<rocket>
Just like with cities and launchers, we will have some wrapper functions. Let's start with the function to update all rockets.
- Create the
UpdateRockets
function. There we loop through therockets
list and call theUpdate
method of each rocket we find.Function UpdateRockets:Int() For Local rocket := Eachin rockets rocket.Update() Next Return True End
The same goes for drawing the rockets on the canvas.
- Add the function
RenderRockets
. Again, it loops through all rockets and calls theRender
method.Function RenderRockets:Int() For Local rocket := Eachin rockets rocket.Render() Next Return True End
To create a rocket, we will have the
CreateRocket
function. - The parameters of the
CreateRocket
function will be the mouse position and the startingx
position.Function CreateRocket:Int(mx:Int, my:Int, xp:Int) Local r:rocket = New rocket 'Create a new rocket r.Init(mx, my, xp) 'Initialize the rocket rockets.AddLast(r) 'Add the rocket to the rockets list Return True End
And, to remove all existing rockets, we have the RemoveRockets function.
- Implement a function called
RemoveRockets
. In it, we call theClear
method of the rockets list.Function RemoveRockets:Int() rockets.Clear() Return True End
The last function we need is one that calculates the distance between two coordinates. We need this to determine speed factors and also for our collision checks later on.
- Create a function called
GetDistance
. The parameters are two pairs ofX
andY
position variables.Function GetDistance:Float( x1:Float, y1:Float, x2:Float, y2:Float ) Local diffx:Float, diffy:Float 'to store the difference diffx = x1 - x2 'Calculate the X difference diffy = y1 - y2 'Calculate the Y difference 'Return the square root of the sum of divX^2 plus divY^2 Return Sqrt((diffx * diffx) + (diffy * diffy)) End
That's all for the wrapper functions. Save the file now. Next stop, the rocket class!
- Create a new class called
rocket
.Class rocket
Now, we will create some data fields to help with animating the rocket.
- First add fields to store the starting position.
Field sx:Float = 0.0 'x start pos Field sy:Float = 0.0 'y start pos
- Next, we need fields to store the target position.
Field tx:Float = 0.0 'x target pos Field ty:Float = 0.0 'y target pos
- The following fields will store the current position.
Field cx:Float = 0.0 'x current pos Field cy:Float = 0.0 'y current pos
- And finally, we add some fields to help with calculating the rocket movement.
Field dx:Float = 0.0 'x difference Field dy:Float = 0.0 'y difference Field speed:Float = 0.0 'speed factor
Ok, we have created the data fields for each rocket. Let's implement some methods.
- Create the
Init
method. The parameters are the target position and the startingx
position.Method Init:Int(rx:Float, ry:Float, xp:Int)
- Next, we will set the starting position.
x
is given by the method parameter andy
is calculated.sx = xp sy = game.cHeight 60.0
- Again, we will store some parameters—the rocket's target position.
tx = rx ty = ry
- Now, we set the current position to the starting position.
cx = sx cy = sy
- To determine difference between the
x
andy
starting position and target, we calculate these by a simple subtraction formula.dx = tx - sx dy = ty - sy
- Lastly, we calculate the speed of the rocket by determining the distance in pixels and dividing it by
4
. Then, close the method.speed = GetDistance(sx, sy, tx, ty)/4.0 Return True End
In each frame, we will need to calculate the new position of a rocket. For this, we need an
Update
method. - Add a method called
Update
.Method Update:Int()
- Calculate the current position by dividing the difference between
x
andy
by the speed factor.cx += dx/speed cy += dy/speed
- Rockets travel upwards, so to check if they have reached their target, you need to check if the current
Y
position is less than the targetY
position.If cy < ty Then
- If the target position is reached, remove the rocket from the
rockets
list. After that, close the IF check, and the method.rockets.Remove(Self) Endif Return True End
Just like cities and launchers, we want to render a rocket.
- Create the
Render
method.Method Render:Int()
- Draw a grey trail behind the rocket with the
DrawLine
statement.SetColor(70, 70, 70) DrawLine(sx, sy, cx, cy)
- Now, draw the rocket head with a random color, and as a circle.
Local cd:Int = Rnd(0, 50) 'Determine a random color factor SetColor(255-cd, 255-cd, 255-cd) DrawCircle(cx, cy, 3)
- Close the method and the class.
Return True End End
What just happened?
That was quick, right? We have a class for our rockets now. We can create them easily and update them through a simple call of a function. Once they reach their target, they destroy themselves.