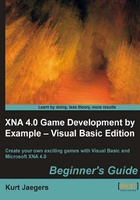
Time for action – GamePiece class methods – part 2 – rotation
- Add the
RotatePiece()
method to theGamePiece
class:Public Sub RotatePiece(clockwise As Boolean) Select Case _pieceType Case "Left,Right" _pieceType = "Top,Bottom" Case "Top,Bottom" _pieceType = "Left,Right" Case "Left,Top" If (clockwise) Then _pieceType = "Top,Right" Else _pieceType = "Bottom,Left" End If Case "Top,Right" If (clockwise) Then _pieceType = "Right,Bottom" Else _pieceType = "Left,Top" End If Case "Right,Bottom" If (clockwise) Then _pieceType = "Bottom,Left" Else _pieceType = "Top,Right" End If Case "Bottom,Left" If clockwise Then _pieceType = "Left,Top" Else _pieceType = "Right,Bottom" End If End Select End Sub
What just happened?
The only information the RotatePiece()
method needs is a rotation direction. For straight pieces, rotation direction does not matter (a left/right piece will always become a top/bottom piece and vice-versa).
For angled pieces, the piece type is updated based on the rotation direction and the previous screenshot.
Tip
Why all the strings?
It would certainly be reasonable to create constants that represent the various piece positions, instead of fully spelling out things, such as Bottom,Left
as strings. However, because the Flood Control game is not taxing on the system, the additional processing time required for string manipulation will not impact the game negatively and helps clarify how the logic works, especially when we get to determine which pipes are filled with water.
Pipe connectors
Our GamePiece
class will need to be able to provide information about the connectors it contains (top, bottom, left, and right) to the rest of the game. Since we have represented the piece types as simple strings, a string comparison will determine what connectors the piece contains.