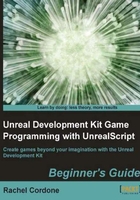
Time for action – Using enums
That's all well and good, but how do we use them? Let's set one up in our AwesomeActor
class.
- Add the enum below our class line.
enum EAltitude { ALT_Underground, ALT_Surface, ALT_Sky, ALT_Space, };
The E isn't necessary, but it helps to follow standard guidelines to make things easier to read.
- Now we need to declare a variable as that enum type. This is similar to declaring other variables.
var EAltitude Altitude;
- Now we have a variable,
Altitude
, that's been declared as the enum typeEAltitude
. Enums default to the first value in the list, so in this case it would beALT_Underground
. Let's see if we can change that in ourPostBeginPlay
function.function PostBeginPlay() { Altitude = ALT_Sky; 'log("Altitude:" @ Altitude); }
Now our class should look like this:
class AwesomeActor extends Actor placeable; enum EAltitude { ALT_Underground, ALT_Surface, ALT_Sky, ALT_Space, }; var EAltitude Altitude; function PostBeginPlay() { Altitude = ALT_Sky; 'log("Altitude:" @ Altitude); } defaultproperties { Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' HiddenGame=True End Object Components.Add(Sprite) }
- Compile and test, and we'll see the enum show up in the log:
[0007.69] ScriptLog: Altitude: ALT_Sky
- To see how they compare to each other, let's add another log after our first one:
'log("Is ALT_Sky greater than ALT_Surface?"); 'log(EAltitude.ALT_Sky > EAltitude.ALT_Surface);
- Doing a comparison like this will come back with a boolean true or false for the answer, which we'll see in the log when we compile and test:
[0007.71] ScriptLog: Altitude: ALT_Sky [0007.71] ScriptLog: Is ALT_Sky greater than ALT_Surface? [0007.71] ScriptLog: True
There we go!
What just happened?
Enums aren't normally something you'd start using when you're new to UnrealScript, but knowing how they work can definitely save time and jumbled code later on. If you were making a card game for example, representing the four different suits as an enum would make it a lot easier to read than if you had numbers representing them. They're not used often, but when they are they're a great help.
Arrays
Now let's take a look at some more complicated uses of variables. Let's say we have several baskets full of kittens, and we wanted to keep track of how many were in each basket. We could make a variable for each basket like this:
var int Basket1; var int Basket2; var int Basket3;
But that would get really messy if we had dozens to keep track of. How about if we put them all on one line like this?
var int Basket1, Basket2, Basket3;
UnrealScript lets us put more than one variable declaration on one line like that, as long as they're the same type. It saves space and keeps things organized. But in this case we'd run into the same problem if we had dozens of baskets. For something like this, we'd need to use an array.