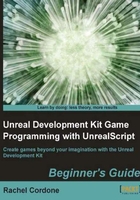
Time for action – Using dynamic arrays
Dynamic arrays are declared a bit differently than static arrays. Let's declare one now.
- Change our variable declaration line to this:
var array<int> Baskets;
As we can see, with dynamic arrays we don't put a number anywhere in it; it can be whatever size we need it to be. By default they're completely empty, so if we tried to log any of its values we would get an out of bounds warning similar to our experiment with static arrays.
- We can, however, assign values any time we want, so let's add this line to our
PostBeginPlay
function:Baskets[3] = 9;
- Then log the value like this:
'log("Baskets:" @ Baskets[3]);
Now our function should look like this:
function PostBeginPlay() { Baskets[3] = 9; 'log("Baskets:" @ Baskets[3]); }
- Compile and test, and we can see that the value logs fine, and we didn't get any warnings.
[0007.82] ScriptLog: Baskets: 9
When we assign a value, the size of the array automatically changes to that value. As with before, if we tried to access
Baskets[4]
we would get an out of bounds warning.Now that we have our dynamic array, there are a few things we need to know about so we can use them properly. The first thing that would be nice to know is the size of the array. Just how many baskets are there anyway? To find out we can use the length of the array.
- Change our log line to look like this:
'log("Basket array length:" @ Baskets.length);
Now our function looks like this:
function PostBeginPlay() { Baskets[3] = 9; 'log("Basket array length:" @ Baskets.length); }
- Compile and test, and check the log file.
[0007.67] ScriptLog: Basket array length: 4
Remember that arrays start out at 0, so when we assigned a value to Basket[3] it's the fourth element in the array.
Now that we know the length of the array, we can use it to add values to the end of it. In our example, the length of the array is 4, with
Baskets[3]
being the last one. If we wanted to add another one to the array, it would beBaskets[4]
. Since 4 is the length of our array right now, we would simply put that in the index. - Let's add these three lines to the end of our function:
Baskets[Baskets.length] = 23; 'log("Basket array length:" @ Baskets.length); 'log("Last basket:" @ Baskets[Baskets.length - 1]);
Our function should look like this now:
function PostBeginPlay() { Baskets[3] = 9; 'log("Basket array length:" @ Baskets.length); Baskets[Baskets.length] = 23; 'log("Basket array length:" @ Baskets.length); 'log("Last basket:" @ Baskets[Baskets.length - 1]); }
The value 23 will now be assigned to the next element in the array, which now expands to five elements.
Remember that the length is one higher than the last index, so to see what the last value is we need to subtract 1 from the array length. In this case the length would be 5, so to check the last one, Basket[4], we need to use 5-1.
- Compile and test, then check the log.
[0008.46] ScriptLog: Basket array length: 4 [0008.46] ScriptLog: Basket array length: 5 [0008.46] ScriptLog: Last basket: 23
And it works! The first line in our function changes the array length to 4 by giving Basket[3] a value of 9. Next we assign a value of 23 to Baskets.length
, which is 4, making Baskets[4] = 23. This also increases the size of the array to 5 which is shown in the log.
What just happened?
Dynamic arrays are very useful when we don't know how many elements we need ahead of time, or the number of elements in the array needs to change during gameplay. As an example, the player's weapons could be held in an array of Actor classes, since they may pick up or lose some during the game.
Have a go hero – Copy an array
Let's say we had an array and PostBeginPlay
set up like this:
var int TestArray[3]; var array<int> CopyArray; function PostBeginPlay() { TestArray[0] = 9; TestArray[1] = 5; TestArray[2] = 6; }
Without using numbers, how would we copy the TestArray
values into the CopyArray
? Think about our experiment on adding to the end of arrays for hints, and when you are ready; check the following code for the answer.
var int TestArray[3]; var array<int> CopyArray; function PostBeginPlay() { TestArray[0] = 9; TestArray[1] = 5; TestArray[2] = 6; CopyArray[CopyArray.length] = TestArray[CopyArray.length – 1]; CopyArray[CopyArray.length] = TestArray[CopyArray.length – 1]; CopyArray[CopyArray.length] = TestArray[CopyArray.length – 1]; }
If you tried TestArray[CopyArray.length]
at first, you probably noticed an out of bounds error in the log. Why is that? Well, an important thing to know about dynamic arrays is that assigning a value to the end of the array increases the size of the array first, before assigning the value. By the time the code reaches the right-hand side of the equals sign, the array's length has already increased by 1.
Structs
The best way to describe a struct would be to call it a variable holding a bunch of other variables. It can be called a collection of variables. As an example, let's take a look at one from Object.uc
:
struct Cylinder { var float Radius, Height; };
As we can see, this struct contains two floats, Radius, and Height. Structs themselves can be used as variables, like this:
var Cylinder MyCylinder;
To change variables inside a struct, we would use a period in between the struct name and the variable name like this:
MyCylinder.Radius = 50;
Structs can contain variables, arrays, and even other structs. Let's take a closer look and make our own struct to learn more about them.