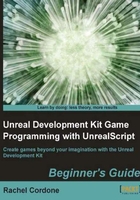
Time for action – Using the default properties block
- Let's start by defining some variables in our
AwesomeActor
class.var string MyName; var int NumberOfKittens; var float DistanceToGo;
- In our default properties block, we can give these variables initial values. These are assigned before any of the code is run.
Defaultproperties { MyName="Rachel" NumberOfKittens=3 DistanceToGo=120.0 }
- In our
PostBeginPlay
function, instead of changing the values we'll just log them to see the default properties in action.function PostBeginPlay() { 'log("MyName:" @ MyName); 'log("NumberOfKittens:" @ NumberOfKittens); 'log("DistanceToGo:" @ DistanceToGo); }
Now our class should look like this:
class AwesomeActor extends Actor placeable; var string MyName; var int NumberOfKittens; var float DistanceToGo; function PostBeginPlay() { 'log("MyName:" @ MyName); 'log("NumberOfKittens:" @ NumberOfKittens); 'log("DistanceToGo:" @ DistanceToGo); } defaultproperties { MyName="Rachel" NumberOfKittens=3 DistanceToGo=120.0 Begin Object Class=SpriteComponent Name=Sprite Sprite=Texture2D'EditorResources.S_NavP' End Object Components.Add(Sprite) }
- Now let's look at the log.
[0008.73] ScriptLog: MyName: Rachel [0008.73] ScriptLog: NumberOfKittens: 3 [0008.73] ScriptLog: DistanceToGo: 120.0000
- Arrays have a slightly different syntax in the default properties. When used in code we use brackets to define the index, like MyArray[2]. In the default properties, we use parentheses instead.
MyArray(0)=3 MyArray(1)=2
You'll also notice that none of the lines in the default properties block have a semicolon at the end. The default properties block is the only place where we don't use them. You'll also notice that there are no spaces before or after the equal sign. This is also a quirk of the default properties block. If the formatting isn't correct you may get a compiler error or even worse, it may compile but ignore your default properties altogether. Make sure to follow these guidelines for the formatting, including having the curly brackets on their own lines. Some programmers like to have the opening curly bracket on the same line, but with UnrealScript's
defaultproperties
block, this would cause it to ignore all of the default properties. - Moving on! For structs, we define the default properties right inside the struct, not in the
defaultproperties
block. So taking our example struct from earlier, we would define the defaults for it like this:struct Basket { var string BasketColor; var int NumberOfKittens, BallsOfString; var float BasketSize; structdefaultproperties { BasketColor="Blue" NumberOfKittens=3 BasketSize=12.0 } };
You may notice that I didn't define a default for BallsOfString
, and that's perfectly fine. Any variable you don't make a default for, will use that variable type's default; in that case BallsOfString
would default to 0.
This is good for structs of our own making, but what about predefined ones like vectors and rotators? Once we declare a variable of those types we can change their default properties in our default properties block, all on the same line. If we had a vector called MyVector
for example, the syntax for the default property would look like this:
MyVector=(X=1.0,Y=5.3,Z=2.1)
This is true for any struct where we've declared a variable of that struct type.
What just happened?
The defaultproperties
block is a convenient place to keep the defaults for our variables, we could use functions like PostBeginPlay
to set values, but it's cleaner and more convenient to have them all in one place. In addition, even if we change the variable during play we can always find out the default value by using this code:
'log(default.BasketSize);
If we wanted to get rid of any changes we've made, we would just reset the variable to its default.
BasketSize = default.BasketSize;